INTRODUCTION
In this tutorial, we are going to learn about the C++98 features elaborately.
C++ is an object-oriented programming language that is a middle-level language. C++ was created, designed, and developed by Bjarne Stroustrup at bell laboratories in new jersey. Earlier, C++ was named C with classes. The danish scientist wanted a flexible and dynamic language that was similar to C with all its features, including active type checking, basic inheritance, default functioning argument, classes, inlining, etc. C with classes was renamed C++ in 1983, resembling one higher level than C.
Below mentioned are some of the major features of the latest version of C++ 98.
- Object-oriented programming
- Machine independent
- Simple
- High-level language
- Popular
- Case sensitive
- Compiler based
- Libraries
- Dynamic memory allocation
- Speed
Object-oriented programming
C++ is an object-oriented programming language whereas C is a procedural language. This is the most important feature of C++. we can create or destroy objects during programming. The concepts of object-oriented programming in C++ are.
- Classes and objects
- Polymorphism
- Encapsulation
- Inheritance
- Abstraction
Machine independent
when the code runs on different platforms operating systems like windows, Linux, macOS, etc, this makes the programming language machine independent. C++ executable file is not platform-independent as the file won’t run on different operating systems. The piece of code which is written in different operating systems makes the C++ language machine independent.
Simple
In C++, we can break the programs into logical units which increases the readability, C++ supports rich libraries and different types of datatypes.
There is also a special keyword used in C++ which is known as auto, which allows us to not define the datatype of the initializer every time. The compiler itself deduces the type of the initializer.
Let’s look at the implementation of the auto keyword with a sample program:
#include <bits/stdc++.h>
using namespace std;
int main()
{
auto integer_val = 26;
auto float_val = 26.24;
cout << typeid(integer_val).name() << "\n";
cout << typeid(float_val).name();
return 0;
}
the output of the program is.
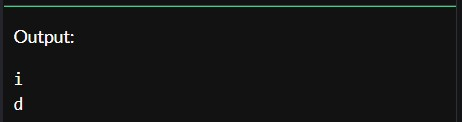
In the above program, we used typeid(parameter). name method to check the datatype of the parameter. i represents integer and d represents double respectively.
High-level language
C++ language is a high-level language as it is closely related to normal human language.
Popular
C++ is one of the most popular programming languages around the world. Due to its advanced features when compared with other programming languages, C++ stands as one of the most popular programming languages. Object-oriented programming is the main feature of C++ which makes the language more popular.
Compiler-based
it is a compiler-based language. The programs in C++ are used to be compiled first and their executable file is used to run it.
Dynamic memory allocation
during the allocation of the variables, the variables are allocated to the heap space. The variables which are present inside the function are allocated to the stack space. This is one of the most useful features of C++.
Allocation of memory during the runtime is known as dynamic memory allocation. In this method, the user doesn’t know the exact size of the memory in advance. Pointers play a major role in dynamic memory allocation.
Let’s understand dynamic memory allocation with a sample program.
#include <iostream>
using namespace std;
int main()
{
double* val = NULL;
val = new double;
*val = 12345.654;
cout << "Value is : " << *val << endl;
delete val;
}
The output of the program is.
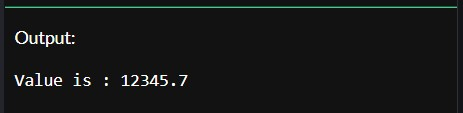
Memory management
C++ allows us to allocate memory for a variable or an array during its runtime. This is known as dynamic memory allocation. In C++, the memory which is allocated must be de-allocated after its usage, this has to be done manually by the user. To allocate and de-allocate memory, we use new and delete operations.
Let’s look at a sample program implementing the usage of new and delete operations.
#include <cstring>
#include <iostream>
using namespace std;
int main()
{
int num = 5;
float* ptr;
ptr = new float[num];
for (int i = 0; i < num; ++i) {
*(ptr + i) = i;
}
cout << "array indexing " << endl;
for (int i = 0; i < num; ++i) {
cout<< i + 1<< " element index : " << *(ptr + i)<< endl;
}
delete[] ptr;
return 0;
}
The output of the program is.
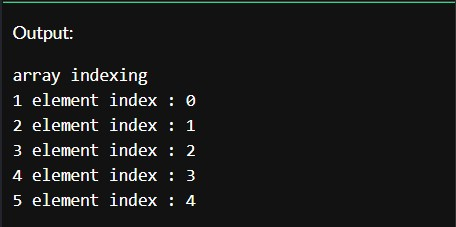
Conclusion
Hurray!. We have learned about the main features of the first version of the C++ language.
If you have any queries leave a comment below. Happy coding!