INTRODUCTION
MULTISET CONTAINER in Cpp
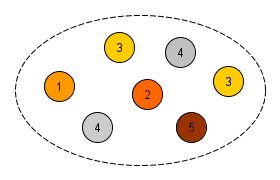
In this tutorial, we are going to learn about the multiset in the standard template library of C++ programming language. Multisets are also similar to normal multisets but in the case of the multiset, it can hold duplicate values. The elements in the multiset can be inserted and deleted but we cannot change the values of the elements in the multiset, they are always constant.
Let’s look at the syntax of the multiset.
multiset < datatype > multiset_name;
Where the datatype represents the data type of the elements stored in the multiset container.
let’s look at some of the basic methods that are implemented on the multiset container.
begin(): this method returns the first element in the multiset.
end(): this method returns the last element in the multiset.
size(): returns the size of the multiset, which means the number of elements in the multiset.
max_size(): this max_size function returns the maximum capacity of elements that a multiset can hold.
empty(): this method returns a boolean value whether the multiset is empty or not.
Insert(const g): this method is used to add a new element ‘g’ to the multiset.
erase(const g): this method is used to remove the element ‘g’ from the multiset.
Some additional methods besides these normal methods are implemented on the multiset. They are.
a.erase(): this method removes all the instances of the element from the multiset which having the same elements.
a.erase(find()): this method removes only one instance of the multiset which has the same elements.
The time complexities of the different operations on the multiset are.
Insertion – O( logN )
Deletion – O( logN )
Searching – O( logN )
Let’s look at some of the properties of the multiset.
- The multiset stores the elements in sorted order.
- All the elements in the set should not be unique likewise sets. Multiple values may or may not be assigned.
- The values of the multiset are immutable. Although we can add or remove values from the multiset, the value of the element cannot be modified once it is added to the multiset.
- Elements stored in multisets don’t have any index values.
Let’s look at a sample program implementing these basic methods on the multiset.
#include <iostream>
#include <iterator>
#include <set>
using namespace std;
int main() {
multiset<int, greater<int> > mulset;
mulset.insert(40);
mulset.insert(30);
mulset.insert(30);
mulset.insert(30);
mulset.insert(60);
mulset.insert(20);
mulset.insert(50);
mulset.insert(50);
mulset.insert(10);
multiset<int, greater<int> >::iterator itr;
cout << "Multiset elements are:\n";
for (itr = mulset.begin(); itr != mulset.end(); ++itr) {
cout << *itr << " ";
}
cout<<endl;
cout<<"\nMultiset after removal of \none instance of 30:\n"<<endl;
mulset.erase(mulset.find(30));
for (itr = mulset.begin(); itr != mulset.end(); ++itr) {
cout << *itr << " ";
}
cout<<endl;
cout << "\nMultiset after removal of \nall instances of 30 :\n";
mulset.erase((30));
for (itr = mulset.begin(); itr != mulset.end(); ++itr) {
cout << *itr << " ";
}
cout<<endl;
cout<<"\nsize of the multiset:\n"<<mulset.size();
return 0;
}
The output of the program is.
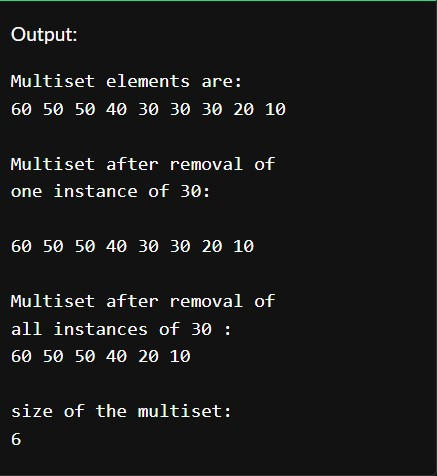
In the above program, we have included the <set> header to create a multiset. Then we created a multiset and inserted the elements in the multiset by using the insert() method. Then we created an iterator to traverse over the multiset container. We have removed one instance of the multiple keys by using the multiset.erase(find()) method. After that, we removed all the instances of the multiple elements of a particular element by using the multiset.erase() method. Then we find the size of the multiset using the size() method. This is the implementation of the basic methods on the multiset.
CONCLUSION
That’s it from this tutorial. We have learned about the multiset and its properties, basic methods implemented on the multiset and a sample program implementing the multiset. Hope you found it interesting. Happy Coding!