Basically, Bank Management Project with python is an intermediate-level project made using Python. Not only a beginner can level up their skill through this project but an intermediate can also sharpen his skills through this. One can even add this amazing project to their portfolio.
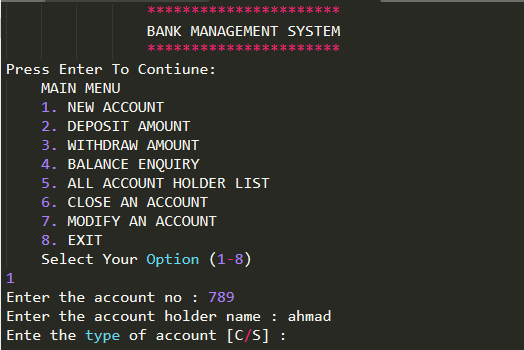
This Bank management project in Python used classes and objects. So if you don’t know about classes in python I will recommend you to check out this post on classes and objects in Python Read Here.
About Bank Management Project with Python
This is a console-based project. All You have to do is run the program and it will open up in the console or terminal depending on whatever IDLE or Code Editor is using. This program is written in classes and objects.
Project Overview: Bank Management Project with Python
Project Name | Bank Management System |
Language | Python |
Python Version | 3.5 + or higher |
IDE or Code editor | Pycharm or Sublimetext3 |
Category | Python Project |
Project Level | Beginner |
Features of this Bank Management Project
Basically, you can have all sorts of functionality that normal banks do have.
- Open a new account
- Deposit amount
- Withdraw Amount
- Balance Enquiry
- All Account Holder List
- Close Bank Account
- Modify Bank Account
Complete Source Code of Bank Management Project
Here is the Complete code of the Bank Management Program using Python.
import pickle
import os
import pathlib
class Account :
accNo = 0
name = ''
deposit=0
type = ''
def createAccount(self):
self.accNo= int(input("Enter the account no : "))
self.name = input("Enter the account holder name : ")
self.type = input("Ente the type of account [C/S] : ")
self.deposit = int(input("Enter The Initial amount(>=500 for Saving and >=1000 for current"))
print("\n\n\nAccount Created")
def showAccount(self):
print("Account Number : ",self.accNo)
print("Account Holder Name : ", self.name)
print("Type of Account",self.type)
print("Balance : ",self.deposit)
def modifyAccount(self):
print("Account Number : ",self.accNo)
self.name = input("Modify Account Holder Name :")
self.type = input("Modify type of Account :")
self.deposit = int(input("Modify Balance :"))
def depositAmount(self,amount):
self.deposit += amount
def withdrawAmount(self,amount):
self.deposit -= amount
def report(self):
print(self.accNo, " ",self.name ," ",self.type," ", self.deposit)
def getAccountNo(self):
return self.accNo
def getAcccountHolderName(self):
return self.name
def getAccountType(self):
return self.type
def getDeposit(self):
return self.deposit
def intro():
print("\t\t\t\t**********************")
print("\t\t\t\tBANK MANAGEMENT SYSTEM")
print("\t\t\t\t**********************")
input("Press Enter To Contiune: ")
def writeAccount():
account = Account()
account.createAccount()
writeAccountsFile(account)
def displayAll():
file = pathlib.Path("accounts.data")
if file.exists ():
infile = open('accounts.data','rb')
mylist = pickle.load(infile)
for item in mylist :
print(item.accNo," ", item.name, " ",item.type, " ",item.deposit )
infile.close()
else :
print("No records to display")
def displaySp(num):
file = pathlib.Path("accounts.data")
if file.exists ():
infile = open('accounts.data','rb')
mylist = pickle.load(infile)
infile.close()
found = False
for item in mylist :
if item.accNo == num :
print("Your account Balance is = ",item.deposit)
found = True
else :
print("No records to Search")
if not found :
print("No existing record with this number")
def depositAndWithdraw(num1,num2):
file = pathlib.Path("accounts.data")
if file.exists ():
infile = open('accounts.data','rb')
mylist = pickle.load(infile)
infile.close()
os.remove('accounts.data')
for item in mylist :
if item.accNo == num1 :
if num2 == 1 :
amount = int(input("Enter the amount to deposit : "))
item.deposit += amount
print("Your account is updted")
elif num2 == 2 :
amount = int(input("Enter the amount to withdraw : "))
if amount <= item.deposit :
item.deposit -=amount
else :
print("You cannot withdraw larger amount")
else :
print("No records to Search")
outfile = open('newaccounts.data','wb')
pickle.dump(mylist, outfile)
outfile.close()
os.rename('newaccounts.data', 'accounts.data')
def deleteAccount(num):
file = pathlib.Path("accounts.data")
if file.exists ():
infile = open('accounts.data','rb')
oldlist = pickle.load(infile)
infile.close()
newlist = []
for item in oldlist :
if item.accNo != num :
newlist.append(item)
os.remove('accounts.data')
outfile = open('newaccounts.data','wb')
pickle.dump(newlist, outfile)
outfile.close()
os.rename('newaccounts.data', 'accounts.data')
def modifyAccount(num):
file = pathlib.Path("accounts.data")
if file.exists ():
infile = open('accounts.data','rb')
oldlist = pickle.load(infile)
infile.close()
os.remove('accounts.data')
for item in oldlist :
if item.accNo == num :
item.name = input("Enter the account holder name : ")
item.type = input("Enter the account Type : ")
item.deposit = int(input("Enter the Amount : "))
outfile = open('newaccounts.data','wb')
pickle.dump(oldlist, outfile)
outfile.close()
os.rename('newaccounts.data', 'accounts.data')
def writeAccountsFile(account) :
file = pathlib.Path("accounts.data")
if file.exists ():
infile = open('accounts.data','rb')
oldlist = pickle.load(infile)
oldlist.append(account)
infile.close()
os.remove('accounts.data')
else :
oldlist = [account]
outfile = open('newaccounts.data','wb')
pickle.dump(oldlist, outfile)
outfile.close()
os.rename('newaccounts.data', 'accounts.data')
# start of the program
ch=''
num=0
intro()
while ch != 8:
#system("cls");
print("\tMAIN MENU")
print("\t1. NEW ACCOUNT")
print("\t2. DEPOSIT AMOUNT")
print("\t3. WITHDRAW AMOUNT")
print("\t4. BALANCE ENQUIRY")
print("\t5. ALL ACCOUNT HOLDER LIST")
print("\t6. CLOSE AN ACCOUNT")
print("\t7. MODIFY AN ACCOUNT")
print("\t8. EXIT")
print("\tSelect Your Option (1-8) ")
ch = input()
#system("cls");
if ch == '1':
writeAccount()
elif ch =='2':
num = int(input("\tEnter The account No. : "))
depositAndWithdraw(num, 1)
elif ch == '3':
num = int(input("\tEnter The account No. : "))
depositAndWithdraw(num, 2)
elif ch == '4':
num = int(input("\tEnter The account No. : "))
displaySp(num)
elif ch == '5':
displayAll();
elif ch == '6':
num =int(input("\tEnter The account No. : "))
deleteAccount(num)
elif ch == '7':
num = int(input("\tEnter The account No. : "))
modifyAccount(num)
elif ch == '8':
print("\tThanks for using bank managemnt system")
break
else :
print("Invalid choice")
ch = input("Enter your choice : ")
FAQs
Question) Is Python used in Finance?
Answer: Yes, in fact, python has become now the most popular programming language in Finance. It’s used by many large corporations, including Google, for a variety of projects.
Question) Is Python the future of Finance and Fintech?
Answer: With the increase in usage of Data Science Applications in Finance sector, the demand for python’s simplicity, popularity, community, and speed will rise.
Hi,I am refering your code for my collage project.But the withdraw money function is not working in the customer section. It’s showing transaction failed. Can you help me.
ok I am updating the code