Introduction:
Python defines an inbuilt module calendar that handles operations related to the calendar. The calendar module allows output calendars like the program and provides additional useful functions related to the calendar. Functions and classes defined in the Calendar module use an idealized calendar, the current Gregorian calendar extended indefinitely in both directions.
Along with the calendar module, we’ll also use the Tkinter module for the GUI of the calendar. I’ll take you through the code line by line for a better understanding of every line of the code.
Project Prerequisites:
- We are only using 2 modules in our project those are the calendar module which is the main module of our project and the Tkinter module for the GUI of our project.
- The calendar module allows you to output the calendars. Before moving to the coding session, we must know what methods we’ll be using for the calendar.
- To know more about the calendar module refer to this link – https://docs.python.org/3/library/calendar.html
- We will also be using the Tkinter module along with the calendar to display the calendar in GUI.
Getting Started:
- As we are using the built-in modules, we don’t have to install any modules.
- First, we’ll start by creating a directory with the name of the project – “Calculator_GUI”.
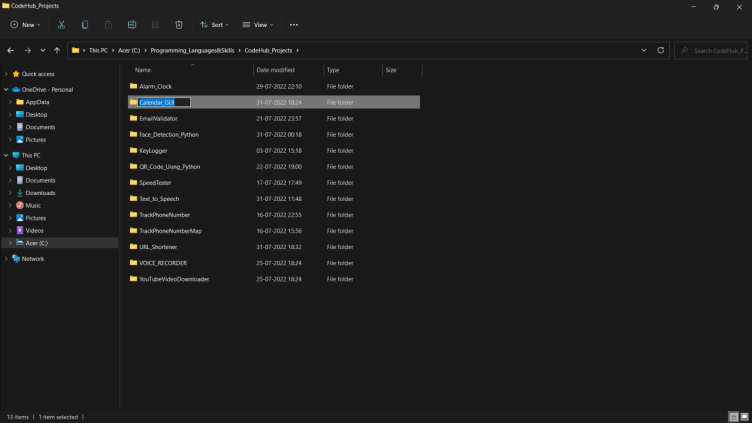
After that, we’ll create a file named – “calendarGUI.py”.

Now that we have completed our setup for the project let’s move to the coding section.
Code Implementation:
You must have good knowledge of the Tkinter module to understand the code better.
We’ll start the implementation by importing the required modules as always.
#Importing tkinter module
from tkinter import *
#importing calendar module
import calendar
#Importing tkinter module
from tkinter import *
#importing calendar module
import calendar
After that, I’ll be defining a function named – “showCalendar”. In this function, we make the GUI for the Calendar and also put the information into the GUI. We run this in a loop so that the GUI stays on the screen.
def showCalender():
gui = Tk()
gui.config(background=’grey’)
gui.title(“Calender for the year”)
gui.geometry(“550×600”)
year = int(year_field.get())
gui_content= calendar.calendar(year)
calYear = Label(gui, text= gui_content, font= “Consolas 10 bold”)
calYear.grid(row=5, column=1,padx=20)
gui.mainloop()
def showCalender():
gui = Tk()
gui.config(background='grey')
gui.title("Calender for the year")
gui.geometry("550x600")
year = int(year_field.get())
gui_content= calendar.calendar(year)
calYear = Label(gui, text= gui_content, font= "Consolas 10 bold")
calYear.grid(row=5, column=1,padx=20)
gui.mainloop()
After defining the method, we will then create an interface layout for displaying the calendar.
new = Tk()
new.config(background=’grey’)
new.title(“Calender”)
new.geometry(“250×140”)
cal = Label(new, text=”Calender”,bg=’grey’,font=(“times”, 28, “bold”))
year = Label(new, text=”Enter year”, bg=’dark grey’)
year_field=Entry(new)
button = Button(new, text=’Show Calender’,fg=’Black’,bg=’Blue’,command=showCalender)
new = Tk()
new.config(background='grey')
new.title("Calender")
new.geometry("250x140")
cal = Label(new, text="Calender",bg='grey',font=("times", 28, "bold"))
year = Label(new, text="Enter year", bg='dark grey')
year_field=Entry(new)
button = Button(new, text='Show Calender',fg='Black',bg='Blue',command=showCalender)
Now, I’ll keep the widgets in the right position that we want.
#putting widgets in position
cal.grid(row=1, column=1)
year.grid(row=2, column=1)
year_field.grid(row=3, column=1)
button.grid(row=4, column=1)
new.mainloop()
#putting widgets in position
cal.grid(row=1, column=1)
year.grid(row=2, column=1)
year_field.grid(row=3, column=1)
button.grid(row=4, column=1)
new.mainloop()
Source Code:
#Importing tkinter module
from tkinter import *
#importing calendar module
import calendar
#function to show calendar of the given year
def showCalender():
gui = Tk()
gui.config(background=’grey’)
gui.title(“Calender for the year”)
gui.geometry(“550×600”)
year = int(year_field.get())
gui_content= calendar.calendar(year)
calYear = Label(gui, text= gui_content, font= “Consolas 10 bold”)
calYear.grid(row=5, column=1,padx=20)
gui.mainloop()
new = Tk()
new.config(background=’grey’)
new.title(“Calender”)
new.geometry(“250×140”)
cal = Label(new, text=”Calender”,bg=’grey’,font=(“times”, 28, “bold”))
year = Label(new, text=”Enter year”, bg=’dark grey’)
year_field=Entry(new)
button = Button(new, text=’Show Calender’,fg=’Black’,bg=’Blue’,command=showCalender)
#putting widgets in position
cal.grid(row=1, column=1)
year.grid(row=2, column=1)
year_field.grid(row=3, column=1)
button.grid(row=4, column=1)
new.mainloop()
#Importing tkinter module
from tkinter import *
#importing calendar module
import calendar
#function to show calendar of the given year
def showCalender():
gui = Tk()
gui.config(background='grey')
gui.title("Calender for the year")
gui.geometry("550x600")
year = int(year_field.get())
gui_content= calendar.calendar(year)
calYear = Label(gui, text= gui_content, font= "Consolas 10 bold")
calYear.grid(row=5, column=1,padx=20)
gui.mainloop()
new = Tk()
new.config(background='grey')
new.title("Calender")
new.geometry("250x140")
cal = Label(new, text="Calender",bg='grey',font=("times", 28, "bold"))
year = Label(new, text="Enter year", bg='dark grey')
year_field=Entry(new)
button = Button(new, text='Show Calender',fg='Black',bg='Blue',command=showCalender)
#putting widgets in position
cal.grid(row=1, column=1)
year.grid(row=2, column=1)
year_field.grid(row=3, column=1)
button.grid(row=4, column=1)
new.mainloop()
Output:
When we run the program. First, a GUI interface will appear asking us to enter the year that we want the calendar for.
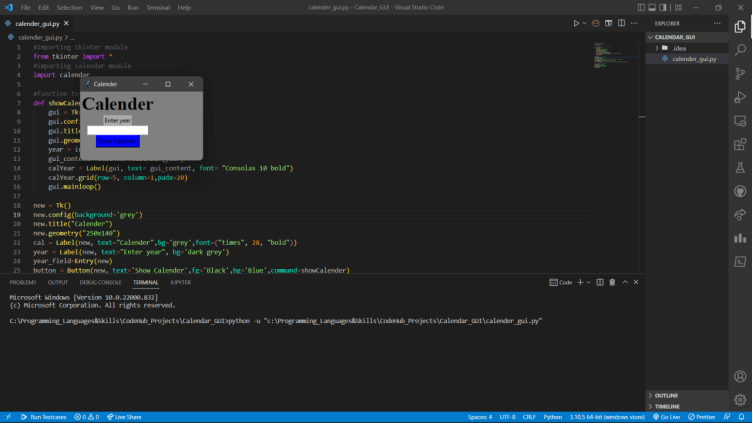
After we have entered the year the calendar corresponding to that year will be displayed in another separate tab.

If you want to know another year’s calendar you can enter that year and that year’s calendar will be displayed.
Congratulations, you now know how to generate a calendar and display using GUI in Python.