In this article, we will learn about the data types in c++.
Data Types
All variables use data types during declaration to restrict the data storage type. So, we can say that data types are used to tell the variables the type of data they can store. Whenever a variable is defined in C++, the compiler allocates some memory for that variable based on the data type it is declared with. Every data type requires a different amount of memory.
C++ supports a wide variety of data types and we can select the data types depending on the need. Data types specify the size and types of values to be stored.
C++ supports the following data types:
- Primary or Built-in or Fundamental data type
- Derived data types
- User-defined data types
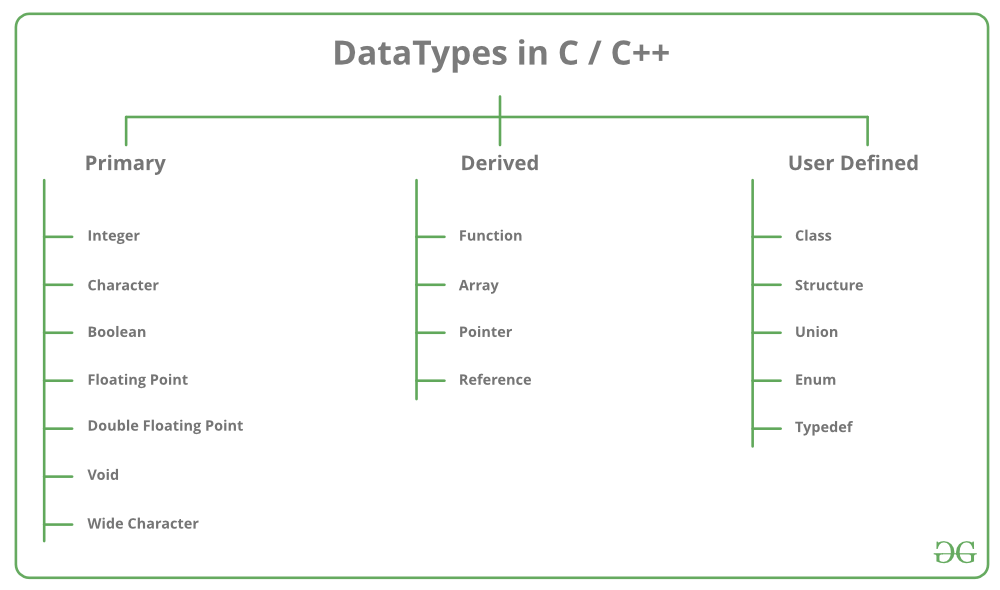
Primitive Data Types:
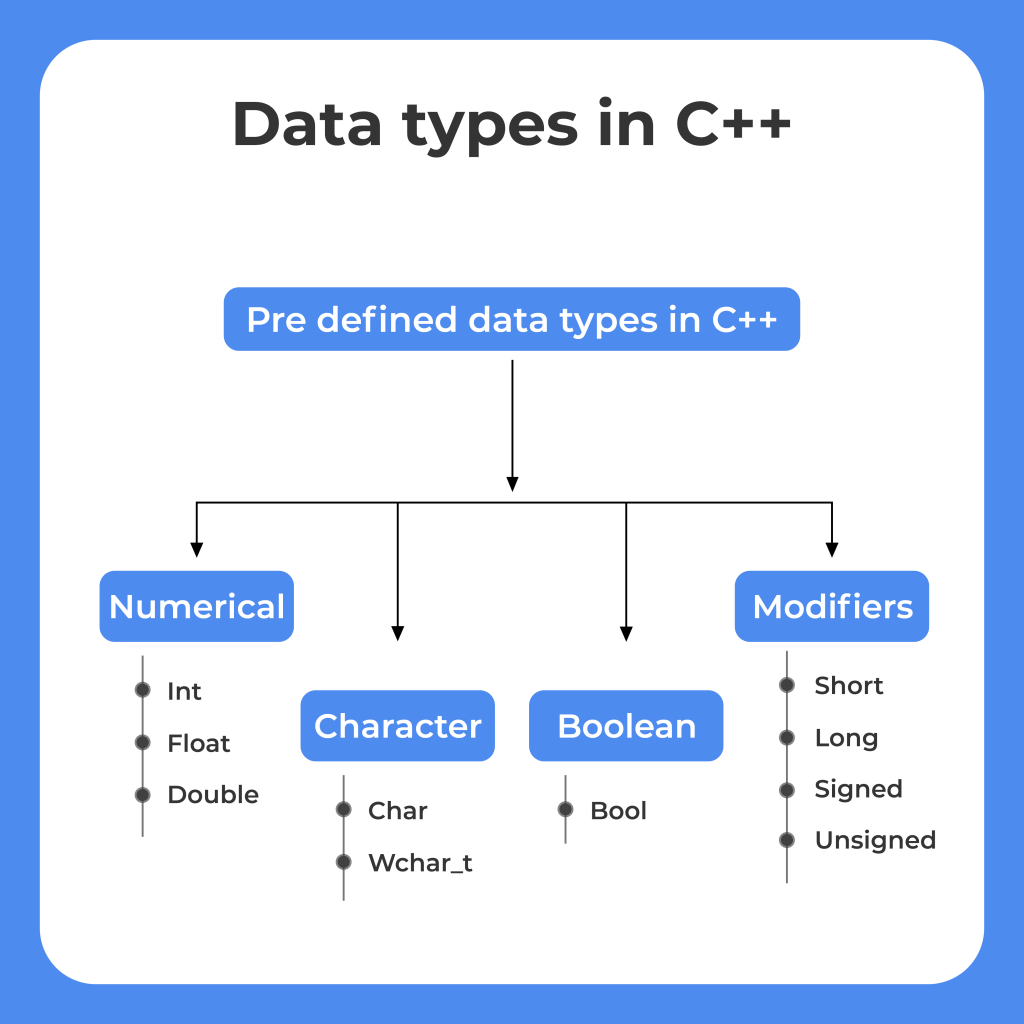
These data types are built-in or predefined data types and can be used directly by the user to declare variables.
Example:
· Integer:
The keyword used for integer data types is int. Integers typically require 4 bytes of memory space and range from -2147483648 to 2147483647.
· Character: Character data type is used for storing characters. The keyword used for the character data type is char. Characters typically require 1 byte of memory space and range from -128 to 127 or 0 to 255.
· Boolean: Boolean data type is used for storing Boolean or logical values. A Boolean variable can store either true or false. The keyword used for the Boolean data type is bool.
· Floating Point: Floating Point data type is used for storing single-precision floating-point values or decimal values. The keyword used for the floating-point data type is float. Float variables typically require 4 bytes of memory space.
· Double Floating Point: Floating Point data type is used for storing single-precision floating-point values or decimal values. The keyword used for the floating-point data type is float. Float variables typically require 4 bytes of memory space.
· Valueless or Void: Void means without any value. void data type represents a valueless entity. A void data type is used for those function which does not return a value.
· Wide Character: Wide character data type is also a character data type but this data type has a size greater than the normal 8-bit data. Represented by wchar_t. It is generally 2 or 4 bytes long.
The size of variables might be different from those mentioned above,
depending on the compiler and the computer you are using.
sizeof() Function:
The sizeof() is used to find the number of bytes occupied by a variable/data type in the computer memory.
Example:
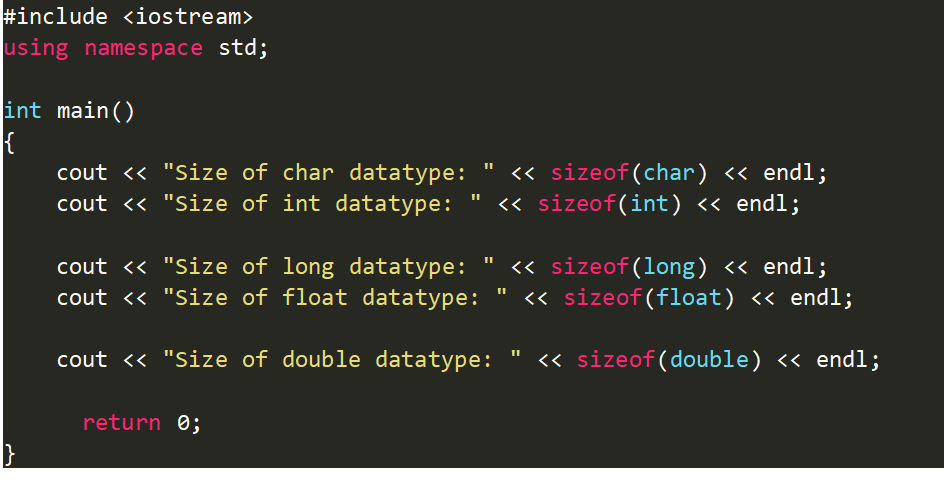
#include <iostream>
using namespace std;
int main()
{
cout << "Size of char datatype: " << sizeof(char) << endl;
cout << "Size of int datatype: " << sizeof(int) << endl;
cout << "Size of long datatype: " << sizeof(long) << endl;
cout << "Size of float datatype: " << sizeof(float) << endl;
cout << "Size of double datatype: " << sizeof(double) << endl;
return 0;
}
Output:
Size of char datatype: 1
Size of int datatype: 4
Size of long datatype: 8
Size of float datatype: 4
Size of double datatype: 8
Derived Data Types:
The data types that are derived from the primitive or built-in datatypes are referred to as Derived Data Types. These can be of four types namely:
- Function
- Array
- Pointer
- Reference
Abstract or User-Defined Data Types:
These data types are defined by the user. Like, class and structure. The following are user-defined data types:
- Class
- Structure
- Union
- Enumeration
- typedef defined Datatype
We will learn about Data type modifiers available in C++ in the next article along with the Macro Constants.
Happy Coding!