In this article, we are going to learn email validation using the python regex module.
Introduction:
An email is a string (a subset of ASCII characters) separated into two parts by @ symbol, a “personal_info” and a domain, that is personal_info@domain. We can use the re (Regular Expression) module in python to do this. A regular expression (or RE) specifies a set of strings that matches it; the functions in this module let you check if a particular string matches a given regular expression (or if a given regular expression matches a particular string, which comes down to the same thing).
Project Prerequisites:
- re module is a built-in module of python that comes along when we install python, so we don’t need to install it separately.
- You should have some prior knowledge about the re (Regular Expression) module.
- To know about the re (Regular Expression) module you can refer to this link –
https://docs.python.org/3/library/re.html
Getting Started:
First, we’ll create a folder for this project named “EMAILVALIDATOR”.
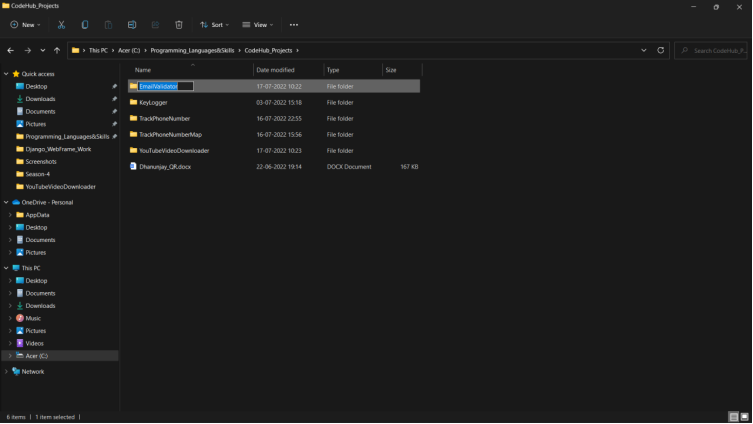
- Then we open that folder in vs-code.
- After, create a python file named “emailvalidator.py”.
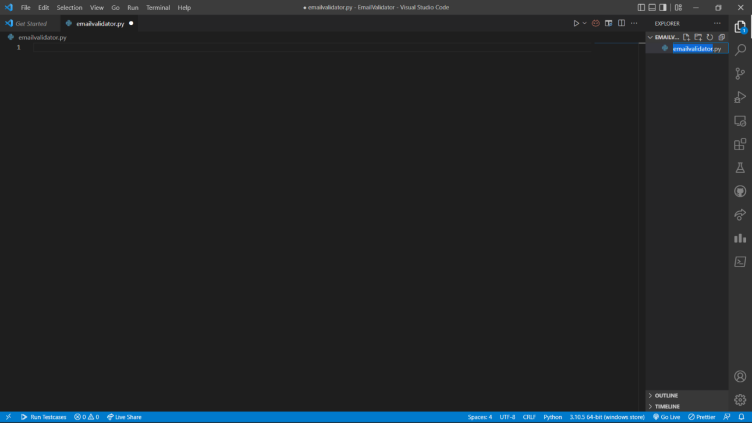
Before we start writing the code, we should know the conditions on how to validate an email. The following are the condition that will be considered during our email validation.
- It should contain alphabets of lowercase only and numeric from 0-9.
- The email has to start with an alphabet and may have any combination of alpha-numeric in it.
- The first part i.e. the part before the @ can have at most 1 character of either “.” (period) (or) “_” (underscore).
- There should be only 1 “@” character in the email.
- After the @ there has to be 1 “.” (period) and its position has to be 2nd or 3rd from the end.
# conditions for a valid email
# a-z lowercase letters only
# 0-9
# . _ anyone at a time
# @ has to be only one
# . has to be 2,3 form end
Code Implementation:
As we are using the re (Regular Expression) module, we’ll be importing it first using the import keyword.
import re
import re
Now we will use Regex to create a raw string with all the conditions of an email that will be used to validate the email we enter.
We can name the variable whatever we want but for understanding, I’ll be naming it as
email_condition.
email_condition = “^[a-z]+[\._]?[a-z 0-9]+[@]\w+[.]\w{2,3}$”
email_condition = "^[a-z]+[\._]?[a-z 0-9]+[@]\w+[.]\w{2,3}$"
We’ll take input of the email from the user
user_email = input(‘Enter user email ID : ‘)
user_email = input('Enter user email ID : ')
Now that we have the email of the user we use the search() method from the re module to validate it. Using if-else we print whether the email is valid or invalid.
if re.search(email_condition,user_email) :
print(‘Valid Email’)
else :
print(‘Invalid Email’)
if re.search(email_condition,user_email) :
print('Valid Email')
else :
print('Invalid Email')
That is all for the code.
Source Code:
Here is the complete source code for the project.
# Python program to validate an Email
# import re module
import re
# Make a regular expression
email_condition="^[a-z]+[\._]?[a-z 0-9]+[@]\w+[.]\w{2,3}$"
# taking input the Email
user_email=input('Enter user email ID : ')
# Validating Email
# We will use the search method from re module
if re.search(email_condition,user_email) :
print('Valid Email')
else :
print('Invalid Email')
Output:
Now that we have completed the code implementation we shall test the project with some Invalid and Valid emails.
First, let us run the code and check for invalid emails.

It’s working perfectly.
Now, let us check for the valid ones.

The code is working just fine.
Congratulations now you know how to validate an email using python.