INTRODUCTION
In this tutorial, we are going to learn about object-oriented programming and its concepts in C++
Firstly, what is object-oriented programming?
Object-oriented programming is the most fundamental programming paradigm used almost everywhere in the programming world. As its name suggests, we use objects in this programming approach. OOPs aims to implement real-life entities like inheritance, data hiding, encapsulation, polymorphism, etc in the programming.
For simple programming tasks, the use of a procedural programming style is well suited. Still, as the program becomes complex and software architecture becomes large, object-oriented programming is suitable to create modular designs and patterns.
C++ supports some of the object-oriented programming concepts below mentioned
- Classes and objects
- Encapsulation
- Data abstraction
- Polymorphism
- Inheritance
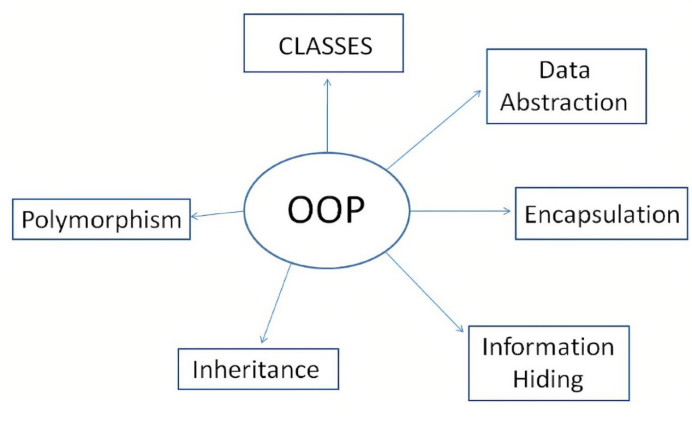
Now we will learn about each concept of object-oriented programming and its uses.
Class:
The classes are the building blocks of object-oriented programming. A class is a user-defined data type, which wraps the data members and functions, which can be accessed and can be used by creating an instance of the class. A class in a blueprint of an object that defines or specifies all the properties of the objects. A class is a physical entity.
For example, consider the class of cars. There may be several cars that exist but all of them share common properties like four wheels, engine, mileage, steering, etc. So, here cars are a class, and wheels, steering, engine, etc are the properties.
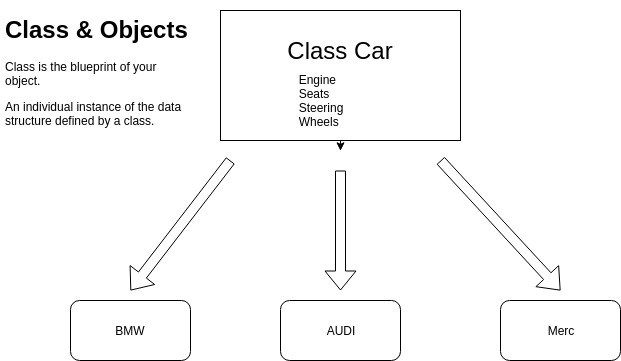
Objects:
Objects are real-life entities with some properties and behavior
. The instance of a class is nothing but an object. No memory is allocated to the class unless an object is instantiated.
Let’s see a sample example of the creation of the objects in C++
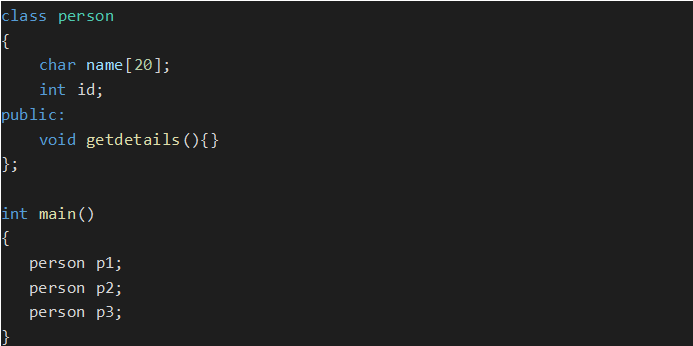
Here, no memory is allocated when the class person is created. But when we created objects named p1, p2, and p3, memory is allocated for each instance of the class. Each object contains data and code to manipulate the data.
Encapsulation:
Encapsulation is nothing but wrapping object state and object behavior in a single unit and protecting them from outside interference. Encapsulation also leads to data abstraction or hiding as it also leads to hiding the data.
There are three basic kinds of encapsulation methods in C++. they are.
Member variable encapsulation: all the data members are declared private in member variable encapsulation. We use getter and setter methods to get and manipulate items in this type of encapsulation.
Function encapsulation: only a subset of member functions are declared private under this kind of encapsulation. The constructor of the class is declared public.
Class encapsulation: all of the classes inside a function are declared private in this type of encapsulation. This type of encapsulation is most basically used in nested classes condition.
Let’s look at a sample program implementing encapsulation.
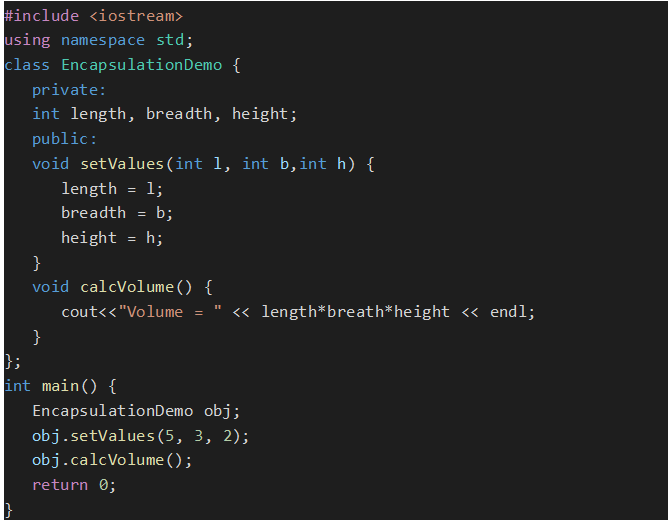
The output of the program is.
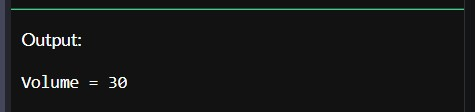
This is an example of class encapsulation. In the above program, all the variables and methods are wrapped in a single unit. All the variables are declared as private and a public function initializes these variables to perform certain tasks. So, this program demonstrates encapsulation.
So, that’s it for this article. In the next article, we will learn about Data abstraction and polymorphism.