In this article, we are going to learn about the pointers in c++.
Pointers are the variables used to store the address of another variable. They are the symbolic representation of the addresses of the variables. However, pointers are considered to be one of the toughest topics to learn in c++. It has many advantages on its way.
Syntax to declare a pointer:
datatype *var_name;
int *ptr; //ptr can point to an address that holds int data

A pointer variable is declared as the following :
datatype *var_name;
The data type is mentioned to get to know about the memory size of the variable which is useful in performing pointer arithmetic(mainly used with arrays)
This is the pictorial representation of how the pointer variable stores the address and is used in referencing and de-referencing a variable.
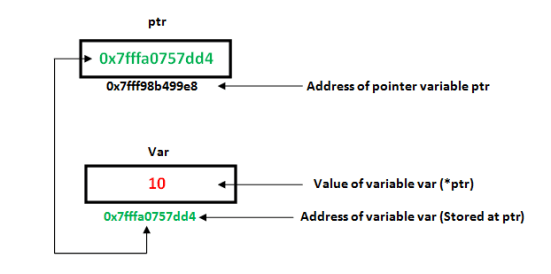
Let’s see how we can implement pointers programmatically:
// C++ program to illustrate Pointers in C++
#include <stdio.h>
void pointersDemo()
{
int var = 20;
// declare pointer variable
int *ptr;
// note that data type of ptr and var must be the same
ptr = &var;
// assign the address of a variable to a pointer
printf("Value at ptr = %p \n",ptr);
printf("Value at var = %d \n",var);
printf("Value at *ptr = %d \n", *ptr);
}
// Driver program
int main()
{
pointersDemo();
}
OUTPUT
Value at ptr = 0x7ffcb9e9ea4c
Value at var = 20
Value at *ptr = 20
We use pointers in c++ to pass variables referring to the functions. Pointers are very useful in passing arguments to functions.
There are 3 ways to pass C++ arguments to a function:
· call-by-value
· call-by-reference with a pointer argument
· call-by-reference with a reference argument
We will learn about these in detail while learning functions in c++.
Array Name as Pointers:
An array name contains the address of the first element of the array which acts like a constant pointer. It means, the address stored in the array name can’t be changed.
For example, if we have an array named val then val and &val[0] can be used interchangeably.
For example, there is an array like the following:
int arr[3] = { 5,10,15};
The memory is allocated like the following:

Pointers Arithmetic & Pointer Expressions:
A limited set of arithmetic operations can be performed on pointers which are:
· incremented ( ++ )
· decremented ( — )
· an integer may be added to a pointer ( + or += )
· an integer may be subtracted from a pointer ( – or -= )
· difference between two pointers (p1-p2)
(Note: Pointer arithmetic is meaningless unless performed on an array.)
// C++ program to illustrate Pointer Arithmetic in C++
#include <bits/stdc++.h>
using namespace std;
void pointersDemo()
{
//Declare an array
int v[3] = {10, 100, 200};
//declare pointer variable
int *ptr;
//Assign the address of v[0] to ptr
ptr = v;
for (int i = 0; i < 3; i++)
{
cout << "Value at ptr = " << ptr << "\n";
cout << "Value at *ptr = " << *ptr << "\n";
// Increment pointer ptr by 1
ptr++;
}
}
//Driver program
int main()
{
pointersDemo();
}
OUTPUT
Value at ptr = 0x7fff9a9e7920
Value at *ptr = 10
Value at ptr = 0x7fff9a9e7924
Value at *ptr = 100
Value at ptr = 0x7fff9a9e7928
Value at *ptr = 200
That’s it for this article. In the next article, we will learn about the
Advanced pointer notations I.e pointers with multidimensional arrays, pointers with string literals, pointers to pointers, types of pointers