In this article, we learn about all the OOP’s concepts (overview).
Introduction:
Python is a multi-paradigm programming language. It supports different programming approaches. One popular approach to solving a programming problem is by creating objects. This is known as Object-Oriented Programming (OOPs). Python has been an object-oriented language since its beginning. It allows us to develop applications with object-oriented approaches. In python, we can easily create and use classes and objects. An object-oriented paradigm is to design the program using classes and objects. The object is related to real-world entities such as books, houses, pencils, etc. the OOP’s concept focuses on writing reusable code. It’s a widespread technique to solve the problem by creating objects. Significant principles of object-oriented programming are given below.
- Class
- Object
- Method
- Inheritance
- Polymorphism
- Data Abstraction
- Encapsulation

Classes & Objects:
Class:
The class can be defined as a collection of objects. A class is a blueprint for the object. It is a logical entity that has some specific attributes and methods. Class logically groups the data in such a way that code re-usability becomes easy. Class is a template or blueprint for how to build an object. A class in python logically grouping of data and functions. An instance is a particular realization of a class. A class is a prototype that defines state and behavior common to all objects of its kind python supports both class objects and instance objects. In fact, everything in python is an object, including a class object. Classes describe data and provide methods to manipulate that data, all encompassed under a single object.
Objects:
An Object contains attributes: data attributes (or static attributes or variables) and dynamic behaviors called methods. In the UML diagram, objects are represented by 3-compartment boxes: name, data attributes, and methods

Methods:
The method is a function that is associated with an object. In python, a method is not unique to the class instance. Any object can have methods
There are three types of methods in python. They are
- Instance method
- Class method
- Static method
Instance method:
an instance method is a very basic and easy method that we use regularly when we create classes in python. we should create an object of the class if we wanted to print the instance attributes or methods.
If we are using self as a function parameter or in front of a variable, that is nothing but the calling instance variable or method itself.
As we are working with instance variables, we use the self keyword.
Note: Instance variables are used with instance methods.
Class method:
There are two ways to create class methods in python:
- Using classmethod(function)
- Using @classmethod annotation
A class method can be called either using the class (such as C.f()) or using an instance (such as C().f()). The instance is ignored except for its class. If a class method is called from a derived class, the derived class object is passed as the implied first argument.
As we are working with ClassMethod we use the cls keyword. Class variables are used with class methods.
Static method:
A static method can be called without an object for that class, using the class name directly. If you want to do something extra with a class, we use static methods.
We can directly call the static method by directly calling it by its class name.
Inheritance:
Inheritance is a mechanism that allows us to inherit all the properties from another class. Acquiring base class properties and methods into a child class is known as inheritance. The main advantage of this is re-usability and increases readability. Python supports single-level, multi-level and multiple inheritances. In python, the object is the parent class of all classes.
It generally means “inheriting or transfer of characteristics from parent to child class without any modification”. The new class is called the derived /child class and the one from which it is derived is called a parent/base class.
Python inheritance super function – super().
With inheritance, the super() function in python actually comes in quite handy. It allows us to call a method from the parent class.
Python super() function provides us the facility to refer to the Parent class explicitly. It is basically useful where we have to call super class functions. It returns the proxy object that allows us to refer to parent class by “super”.
Different forms of inheritances:
- Single level inheritance
- Multi-level inheritance
- Multiple inheritance
- Hierarchical inheritance
Polymorphism:
Polymorphism in python is used for a common function name that can be used for different types. In python, we can find the same operator or function taking multiple forms. It is also useful in creating different classes which will have methods having the same name. The main use of polymorphism is decreasing the code length and complexity by re-using the code.
Polymorphism can be done in two ways.
- Method overriding
- Operator overloading
Method overriding:
method overriding occurs when there are two methods with the same name and with the same return type in the same class. method overriding also occurs when there also exists a method with the same name both in the parent class and child class. Method overriding is an ability of any object-oriented programming language that allows a subclass or child class to provide a specific implementation of a method that is already provided by one of its super-classes.
Overriding is a feature of using the functions of parent class into child class to increase the readability.
Sample program:

Output:

Operator overloading:
operator overloading in python shows different behaviour for objects of different classes, this is called operator overloading. This operator overloading can be clearly understood by the following example.
Sample program:
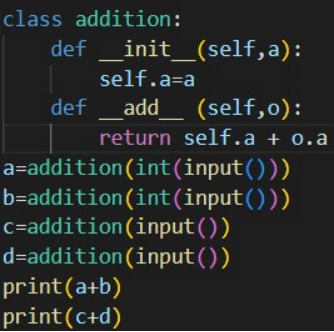
Output:

In the above program, the magic method __add__ is automatically invoked in which the operation for + operator is defined.
Data abstraction:
In the process of abstraction in Python, a programmer can hide all the irrelevant data of an application to reduce complexity and increase efficiency. data abstraction means making the data private so that the complexity of the code will be reduced and the efficiency of the code will be increased. In python, if we want to hide a variable, then we need to write double underscore “ __ “before the variable name, so that the variables will not be directly visible outside.
Advantages:
- Enhancement of the security against hackers
- Isolation of the objects from the basic OOP
- High security which stops damage to violate data by hiding it from public
Disadvantages:
- length of the code will be increased sometimes accordingly
- The more you Increase the layers of abstraction, the less will be the efficiency of the code.
Encapsulation:
Encapsulation is one of the fundamental concepts in object-oriented programming (OOP). Encapsulation describes the idea of wrapping data and the methods that work on data within one unit. This puts restrictions on accessing variables and methods directly and can prevent the accidental modification of data. To prevent accidental change, an object’s variable can only be changed by an object’s method. Those types of variables are known as private variables.
A class is an example of encapsulation as it encapsulates all the data that is member functions, variables, etc.
Advantages:
- Protection of data from unauthorized access
- Increases the code readability and maintainability by building data and methods within a class
- Helps in prevention of accidental data modification
Disadvantages:
- Increased execution of code time
- Length of the code increases drastically in some cases