Introduction:
In the previous article, we learned about inheritance. so let’s learn about the types of inheritance with example programs.
Types of inheritance:
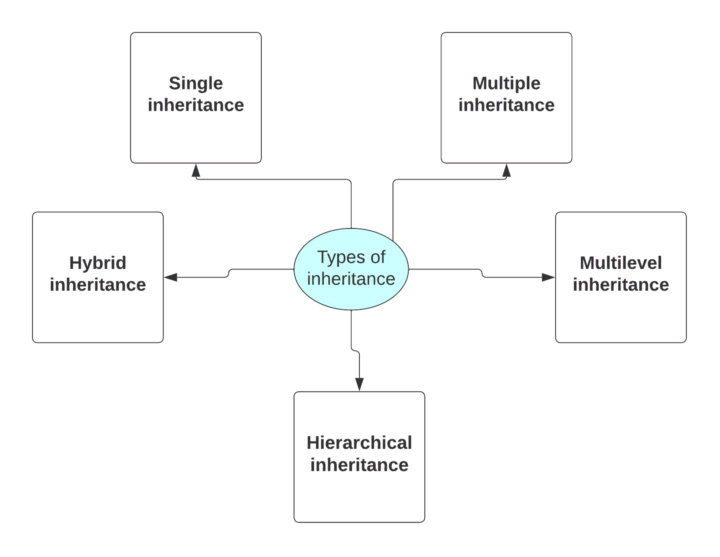
1. Single Inheritance:
single inheritance is defined as the inheritance in which a derived class is inherited from only one base class.
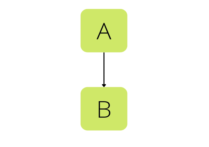
syntax:
class subclass_name : access_mode base_class
class A
{
... .. ...
};
class B: public A
{
... .. ...
};
Program:
#include <iostream>
using namespace std;
class Account {
public:
float salary = 60000;
};
class Programmer: public Account {
public:
float bonus = 5000;
};
int main(void) {
Programmer p1;
cout<<"Salary: "<<p1.salary<<endl;
cout<<"Bonus: "<<p1.bonus<<endl;
return 0;
}
Output:
Salary: 60000
Bonus: 5000
2. Multilevel Inheritance:
In this type of inheritance, a derived class is created from another derived class.
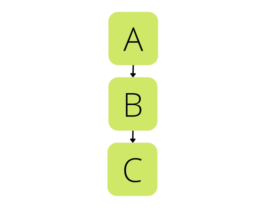
syntax:
class C
{
... .. ...
};
class B: public C
{
... .. ...
};
class A: public B
{
... ... ...
};
Program:
#include <iostream>
using namespace std;
// base class
class Vehicle {
public:
Vehicle() { cout << "This is a Vehicle\n"; }
};
// first sub_class derived from the class vehicle
class fourWheeler: public Vehicle {
public:
fourWheeler()
{
cout << "Objects with 4 wheels are vehicles\n";
}
};
// subclass derived from the derived base class four wheeler
class Car : public fourWheeler {
public:
Car() { cout << "Th car has 4 Wheels\n"; }
};
// main function
int main()
{
// Creating object of subclass will
// invoke the constructor of base classes.
Car obj;
return 0;
}
output:
This is a Vehicle
Objects with 4 wheels are vehicles
The car has 4 Wheels
3. Multiple Inheritance:
Multiple Inheritance is a feature of C++. A class can inherit from more than one class. one class can be inherited from more than one base class.
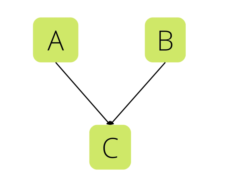
syntax:
class subclass_name : access_mode base_class1, access_mode base_class2, ....
{
// body of subclass
};
class B
{
... .. ...
};
class C
{
... .. ...
};
class A: public B, public C
{
... ... ...
};
The access mode for every base class must be specified.
Program:
// C++ program to explain
// multiple inheritance
#include <iostream>
using namespace std;
// first base class
class Vehicle {
public:
Vehicle() { cout << "This is a Vehicle\n"; }
};
// second base class
class FourWheeler {
public:
FourWheeler()
{
cout << "This is a 4-wheeler Vehicle\n";
}
};
// subclass derived from two base classes
class Car : public Vehicle, public FourWheeler {
};
// main function
int main()
{
// Creating object of subclass will
// invoke the constructor of base classes.
Car obj;
return 0;
}
Output:
This is a Vehicle
This is a 4-wheeler Vehicle
4. Hierarchical Inheritance:
In this type of inheritance, more than one subclass is inherited from a single base class. i.e. more than one derived class is created from a single base class.
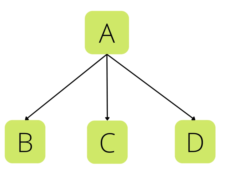
syntax:
class A
{
// body of class A.
}
class B : public A
{
// body of class B.
}
class C : public A
{
// body of class C.
}
class D : public A
{
// body of class D.
}
Program:
#include <iostream>
using namespace std;
// base class
class Vehicle {
public:
Vehicle() { cout << "This is a Vehicle\n"; }
};
// first sub class
class Car : public Vehicle {
};
// second subclass
class Bus : public Vehicle {
};
int main()
{
// Creating object of sub-class will
// invoke the constructor of the base class.
Car obj1;
Bus obj2;
return 0;
}
Output:
This is a Vehicle
This is a Vehicle
5. Hybrid Inheritance:
Hybrid Inheritance is implemented by combining more than one type of inheritance. For example: Combining Hierarchical inheritance and Multiple Inheritance.
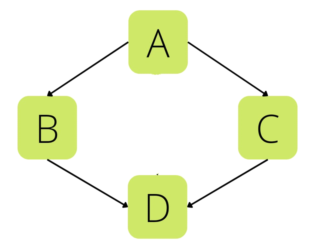
Program:
#include <iostream>
using namespace std;
// base class
class Vehicle {
public:
Vehicle() { cout << "This is a Vehicle\n"; }
};
// base class
class Fare {
public:
Fare() { cout << "Fare of Vehicle\n"; }
};
// first sub class
class Car : public Vehicle {
};
// second sub class
class Bus : public Vehicle, public Fare {
};
// main function
int main()
{
// Creating object of sub-class will
// invoke the constructor of the base class.
Bus obj2;
return 0;
}
Output:
This is a Vehicle
Fare of Vehicle