In the next 3 articles, we are going to learn about the decision-making statements in c++.
Decision Making Statements
The decision-making statements are also known as conditional statements. There are situations in life when we have to make decisions and based on that we decide what we do next. Similarly, that kind of situation may occur in programming, we will make some decisions based on the conditions and execute a certain block under that condition. There can also be multiple conditions like if a certain condition satisfies, we execute a block and if not, we check for another condition and execute that block if that condition is satisfied, and if none of the conditions are satisfied then a block is executed that the remaining part of the code is executed normally.
Types of Decision-Making Statements
The below picture shows the different types of decision-making statements in C++.

The decision-making statements in C++ or any other programming language decide the flow of the program execution.
- if statement
- if…else statements
- nested if statements
- if-else-if ladder
- switch statements
- Jump Statements:
- break
- continue
- goto
- return
NOTE: In this article, you will be learning about the if, if…else, nested if, and if-else-if ladder. The switch and Jump statements will be covered in the next article along with a small application program (Simple Calculator).
if Statement
The if statement is the simplest decision-making statement. It is used to decide whether or not to execute a certain line or block of code. If the given condition is satisfied then the line or block of code inside the if statement is executed otherwise not.
The if statement is the simplest decision-making statement. It is used to decide whether or not to execute a certain line or block of code. If the given condition is satisfied then the line or block of code inside the if statement is executed otherwise not.
Syntax:
if(condition)
{
// Statements to execute if
// condition is true
}
Here the condition after evaluation will be either true or false. The curly braces ‘{‘ and ‘}’ are given in order to execute all the statements inside them if the condition is true, if they were not given then the statement just below the if statement is considered to be inside the block.
Example:
if(condition)
statement1;
statement2;
// Here if the condition is true, if block
// will consider only statement1 to be inside
// its block.
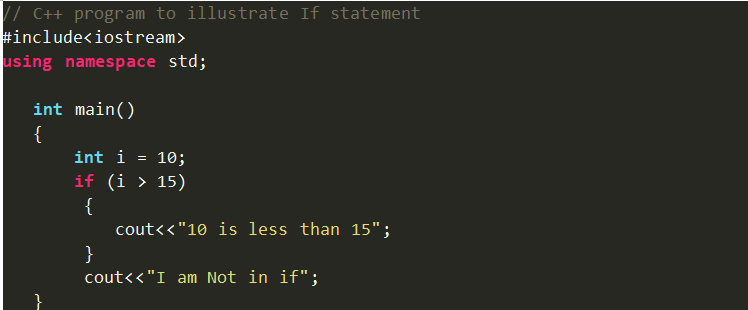
// C++ program to illustrate If statement
#include<iostream>
using namespace std;
int main()
{
int i = 10;
if (i > 15)
{
cout<<"10 is less than 15";
}
cout<<"I am Not in if";
}
Output:
I am Not in if
As the condition present in the if statement is evaluated to false, the block below the if statement is not executed.
Flow Chart:
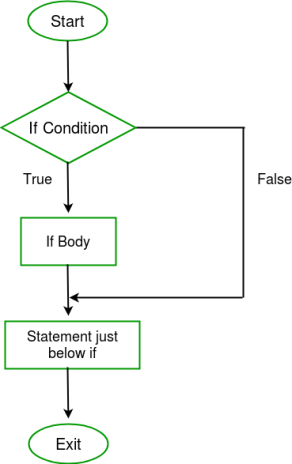
if-else Statements
We have seen how the if statement works. Now, the if-else statement also works the same way but for one thing that is if the condition is evaluated to false in the if statement there is nothing specific to do, so the next statements are executed but, in the if-else statements if the condition is evaluated to false the else statement block is executed and then the normal flow of execution continues.
Syntax:
if (condition)
{
// Executes this block if
// condition is true
}
else
{
// Executes this block if
// condition is false
}
Example:
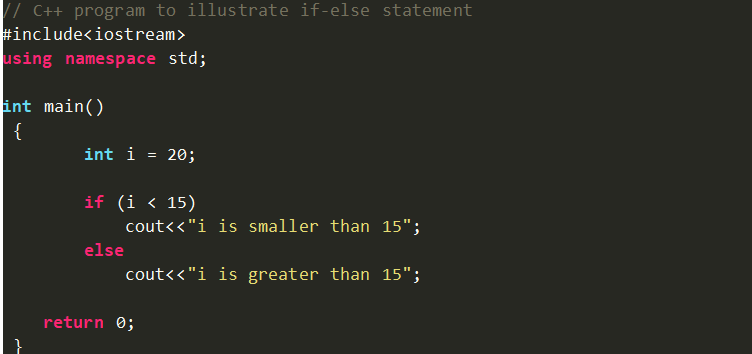
// C++ program to illustrate if-else statement
#include<iostream>
using namespace std;
int main()
{
int i = 20;
if (i < 15)
cout<<"i is smaller than 15";
else
cout<<"i is greater than 15";
return 0;
}
Output:
i is greater than 15
The block of code following the else statement is executed as the condition present in the if statement is false.
Flow Chart:
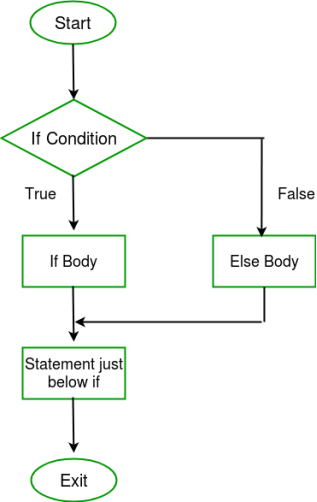
Nested if-else
A nested if-else in C++ is an if-else statement that is the target of another if-else statement. Nested if-else statements mean an if-else statement inside another if or else statement. We can use nested if statements within if statements, i.e, we can place an if statement inside another if statement.
Syntax:
if (condition1)
{
// Executes when condition1 is true
if (condition2)
{
// Executes when condition2 is true
}
}
Example:
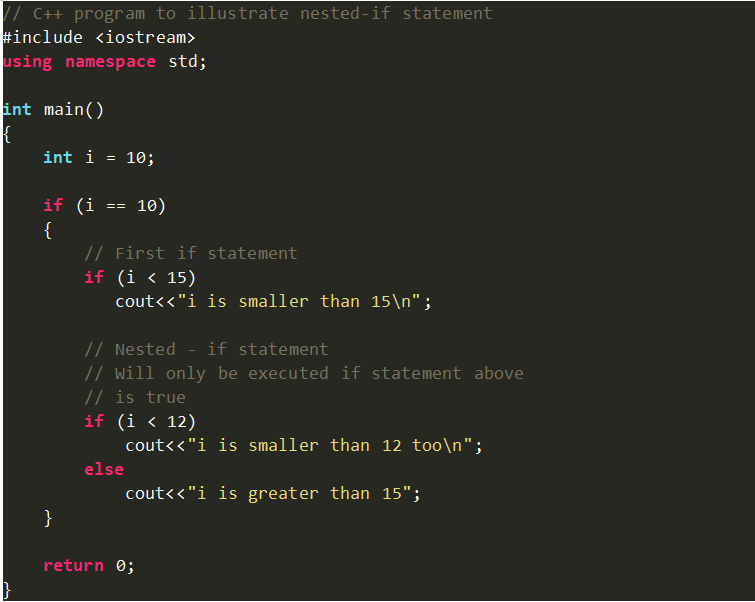
/ C++ program to illustrate nested-if statement
#include <iostream>
using namespace std;
int main()
{
int i = 10;
if (i == 10)
{
// First if statement
if (i < 15)
cout<<"i is smaller than 15\n";
// Nested - if statement
// Will only be executed if statement above
// is true
if (i < 12)
cout<<"i is smaller than 12 too\n";
else
cout<<"i is greater than 15";
}
return 0;
}
Output:
i is smaller than 15
i is smaller than 12 too
Flow Chart:
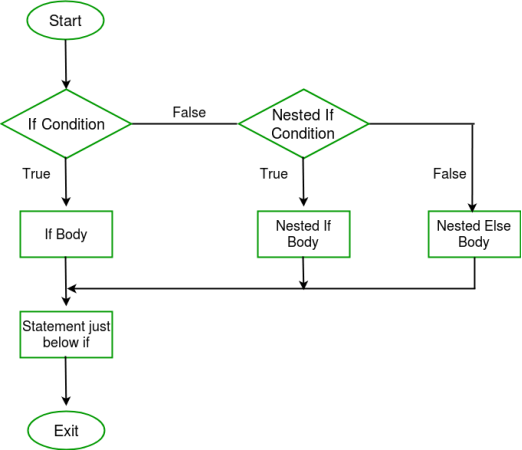
if & else-if ladder
Until now we have seen how can we check a certain condition and make decisions accordingly but what if we want to check multiple conditions and execute that block whose condition is satisfied, that is where the if-else-if ladder comes into play. Using the if-else-if ladder we can check for multiple conditions and execute the block where the condition is satisfied.
Syntax:
if (condition)
statement;
else if (condition)
statement;
.
.
else
statement;
Example:
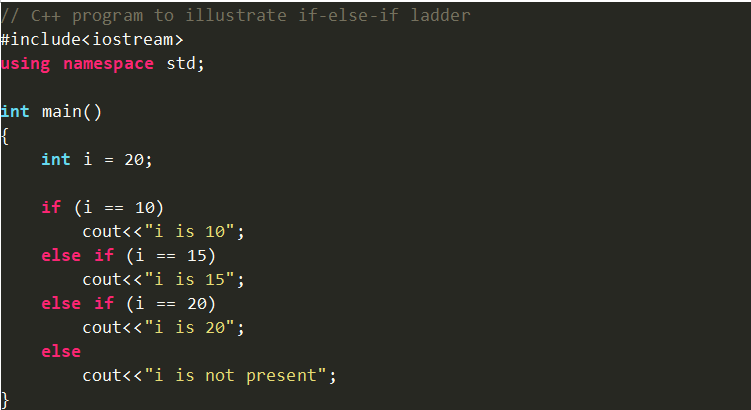
// C++ program to illustrate if-else-if ladder
#include<iostream>
using namespace std;
int main()
{
int i = 20;
if (i == 10)
cout<<"i is 10";
else if (i == 15)
cout<<"i is 15";
else if (i == 20)
cout<<"i is 20";
else
cout<<"i is not present";
}
Output:
i is 20
Flow Chart:
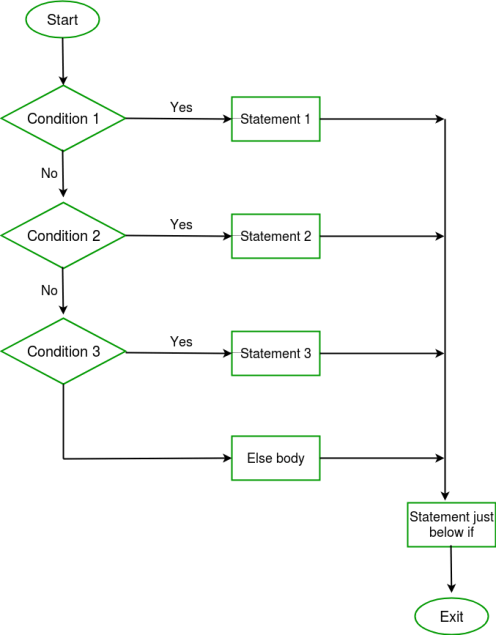
That is all for this article. Hope you understood the decision-making statements (if, if…else, nested if, and if-else-if ladder).
Happy Coding!