INTRODUCTION
MULTIMAP CONTAINER in Cpp
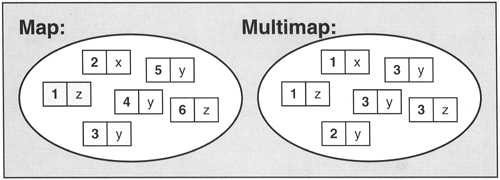
In this tutorial, we are going to learn about multimap containers from the standard template library of C++ programming language. A multimap is an associative container that is similar to a normal map. The multimap is used to store multiple non-unique values to the same key value. This is the only exception that makes difference between a normal map and a multimap.
Let’s look at the syntax of the multimap.
multimap <key_value datatype , mapped_value datatype> multimap_name;
Where the key_value data type and mapped_value data type represent the datatypes of the key and the mapped value respectively.
Let’s look at some of the properties of the multimap.
The multimap map is similar to a normal map. The thing is, a multimap can store multiple elements with the same key value.
The keys in the multimap are always stored in sorted order. This reflects the storage of the respective mapped values associated with the key values.
The time complexity for different basic operations like searching, inserting and deleting elements is O( logN ).
These are some of the basic properties of the multimap container.
Let’s look at some of the basic methods that are applied on the multimap.
insert(): this method adds a new item to the multimap in a specific order
erase(): this method removes the particular item from the multimap.
begin(): this method returns an iterator to the first element in the multimap.
end(): this method returns an iterator to the last element in the multimap.
size(): this method is used to return the number of items stored in the multimap.
max_size(): this method returns the maximum number of elements that a multimap can hold.
empty(): this method returns a boolean value whether the particular multimap is empty or not.
clear(): this method is used to remove all the elements from the multimap and empty it.
Let’s look at a sample program implementing the creation of the multimap container.
#include <iostream>
#include <iterator>
#include <map>
using namespace std;
int main() {
multimap<int, int> m;
m.insert(pair<int, int>(1, 40));
m.insert(pair<int, int>(2, 30));
m.insert(pair<int, int>(3, 60));
m.insert(pair<int, int>(6, 50));
m.insert(pair<int, int>(6, 10));
multimap<int, int>::iterator itr;
cout << "Multimap is: \n";
for (itr = m.begin(); itr != m.end(); ++itr) {
cout<<(*itr).first<<"\t"<<(*itr).second<<endl;;
}
m.insert(pair<int, int>(4, 50));
m.insert(pair<int, int>(5, 10));
cout << "Multimap after inserting elements: \n";
for (itr = m.begin(); itr != m.end(); ++itr) {
cout<<(*itr).first<<"\t"<<(*itr).second<<endl;
}
cout << endl;
return 0;
}
The output of the program is.
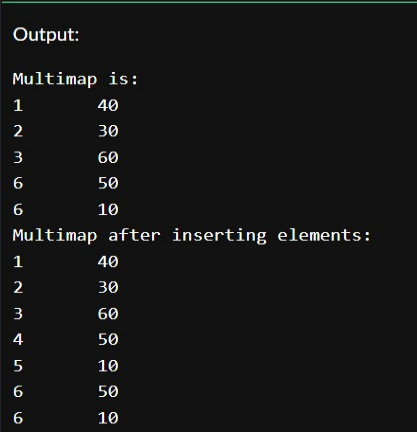
In the above program, we have included the < map > header to create a multimap. After creating a multimap, we inserted the elements into the array using the insert() function. After inserting the items, we created an iterator to traverse over the multimap. This is the implementation of the multimap container.
Let’s look at another program implementing the basic methods on the multimap.
#include <iostream>
#include <iterator>
#include <map>
using namespace std;
int main() {
multimap<int, int> m;
m.insert(pair<int, int>(1, 40));
m.insert(pair<int, int>(2, 30));
m.insert(pair<int, int>(3, 60));
m.insert(pair<int, int>(6, 50));
m.insert(pair<int, int>(6, 10));
multimap<int, int>::iterator itr;
cout<<"Size of the multimap is: \n"<<m.size()<<endl<<endl;
if(m.empty())
cout<<"multimap is empty\n";
else
cout<<"multimap is not empty\n";
m.erase(6);
cout<<"\nMultimap after removing items with\nkey value 6:\n";
for (itr = m.begin(); itr != m.end(); ++itr)
cout<<(*itr).first<<"\t"<<(*itr).second<<endl;
return 0;
}
The output of the program is.
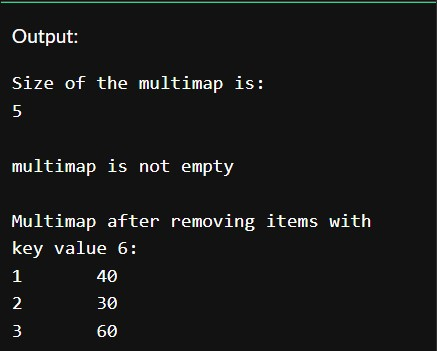
In the above program, the count of the number of elements in the multimap is calculated by using the size() method. We checked whether the multimap is empty or not by using the empty() method. After that, we removed the items associated with the key value 6 by using the erase() method. This is the implementation of the basic methods on the multimap.
Let’s see the differences between a normal map and a multimap.
MAP | MULTIMAP |
The map stores unique key-value pair where each key is unique. | The multimap stores non-unique values for the same key. |
The elements of the map are directly accessible and accessing is easy, | The elements of the multimap cannot be directly accessed and accessing is not that easy. |
The erase() function removes only one item with the respective key. | The erase() function removes all the items of the respective key. |
The count() method returns binary values. | The count() method returns any non-negative key. |
CONCLUSION
That’s it from this tutorial. We have learned about the multimap container, its properties, basic methods that are implemented on multimaps and differences between the maps and the multimaps. Hope you guys found it interesting. Happy Coding!