INTRODUCTION
In this tutorial, we will learn about the constructor in C++ and its purposes
Firstly, what is a constructor?
In object-oriented programming, the constructor is a member method with no return values and return types, which is used to create objects of the class. It is a special method which is invoked automatically at the time of object creation. In C++, the name of the constructor is the same as the name of the class. The name itself depicts that the constructor provides the data for the objects, that’s why it is named the constructor.
Let’s look at the syntax of the constructor in C++.
The constructor may be defined within the class or outside the class. The related syntax of the definition of the constructor is given as.
Within the class:
class-name (list-of-parameters)
{
// constructor definition
}
class-name (list-of-parameters)
{
// constructor definition
}
Outside the class:
class-name: :class-name (list-of-parameters)
{
// constructor definition
}
class-name: :class-name (list-of-parameters)
{
// constructor definition
}
We knew that there are some differences between the constructor of the class and the normal methods defined in the class. What are the actual properties that differentiate the constructor from the normal methods? What is the functionality that makes the constructor differ from the other methods? Let’s take a look at the differences between the constructor and the normal methods.
DIFFERENCES BETWEEN CONSTRUCTOR AND NORMAL METHODS
methods | constructor |
Methods get executed when we call them explicitly. | Constructor gets executed when an object is created. |
We can give any name to the method based on its functionality and its use | The name of the constructor should be always the name of the class. |
The methods may or may not have a return type. | The constructor doesn’t have a return type. |
A method can be executed many times based on preference. | The constructor gets executed only once per object creation. |
In C++, there are three types of constructors. They are.
- Default constructor
- Parameterized constructor
- Copy constructor
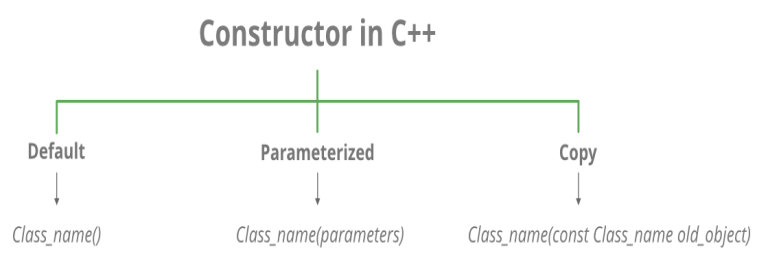
Default constructor
The default constructor is also known as the zero-argument constructor, which doesn’t have any parameters. The default constructor doesn’t take any kind of argument.
Let’s look at the syntax of the default constructor
class-name ( )
{
// constructor definition
}
Let’s look at a sample program implementing the default constructor
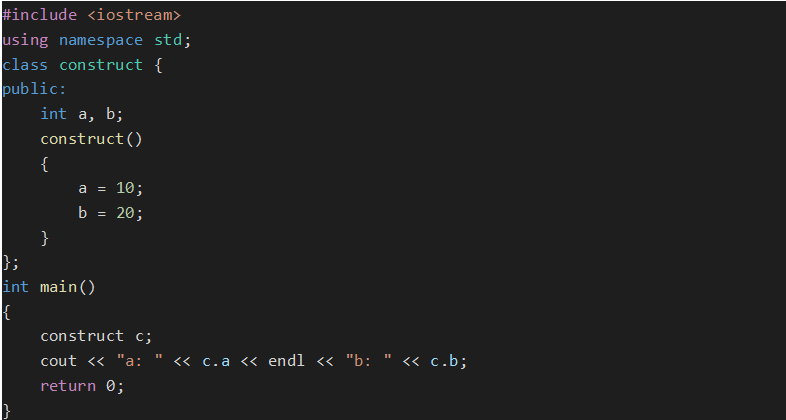
The output of the program is.
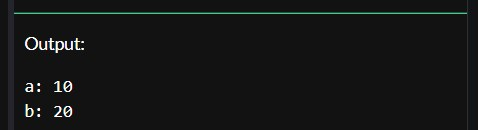
In the above example, we named a constructor with the class name itself, without passing any kind of arguments. Later by using the constructor, we created an object and accessed the values of the object.
Parameterized constructor
In the parameterized constructor, we can pass any kind of argument to the constructor. The arguments passed in the constructor are used to initialize an object when it is created. The parameterized constructor is as similar to the default constructor, with the arguments passed.
Let’s look at the syntax of the parameterized constructor.
class-name ( list of parameters )
{
// constructor definition
}
Let’s look at a sample program implementing parameterized constructor.
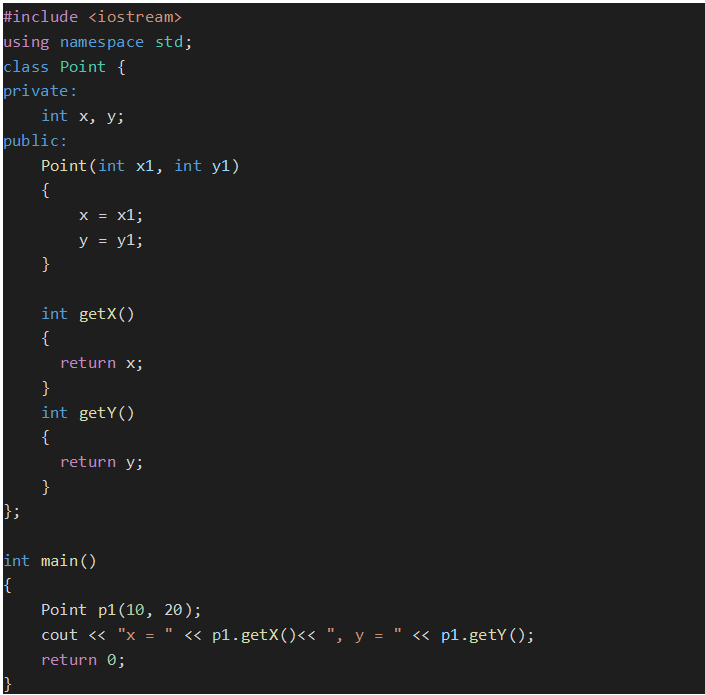
The output of the program is.
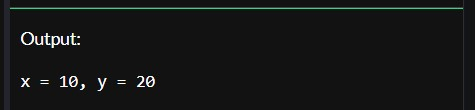
Copy constructor
The copy constructor is a kind of constructor which initializes an object by using another object of the same class. We can initialize values to the new object by using the existing object. This method of initialization of values to the new object by using the old object by using copy constructor is known as copy initialization.
Let’s look at a sample program implementing copy constructor.
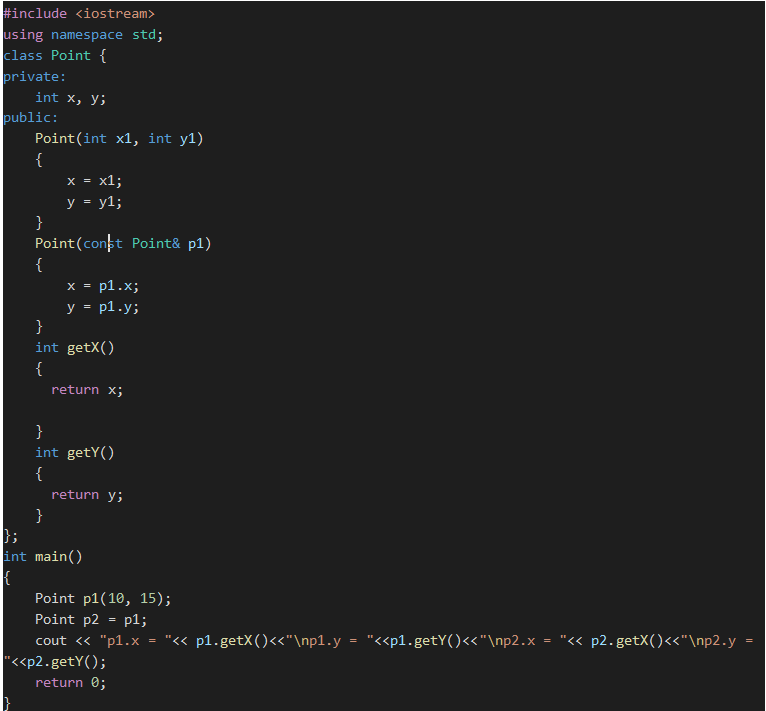
The output of the program is.
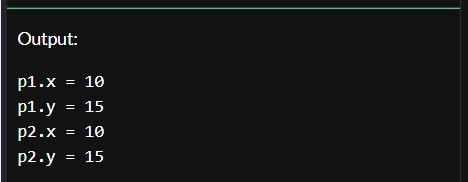
CONCLUSION
That’s it from this tutorial. We have learned about constructor, its functionality, differences between constructor and normal methods and different types of constructors. Hope you find it interesting. Happy coding!