INTRODUCTION
In this tutorial, we are going to learn about the destructors in C++. so, we learned about the constructors and their different types. So, what is a destructor?
The constructor is a member function that is invoked automatically when an object is created. Similarly, a destructor is an instance member function that is invoked automatically when an object is going to be destroyed. We can simply define destructor as the last function which is called before an object of a class is going to be destroyed.
We can define a destructor within a class or outside of the class.
The syntax of defining a destructor inside a class is as follows.
~ <class-name>()
{
// body of the destructor
}
The syntax for defining a destructor outside a class is as follows.
<class-name>: : ~ <class-name>()
{
// body of the destructor
}
here, a special symbol titled symbol ( ~ ) is used which is used to create a destructor. The name of the destructor is as same as the name of the class name followed by the titled symbol.
Let’s look at some of the key points to be noted about a destructor.
- Every class can have only one destructor. It is not possible to define more than one destructor.
- If you want to delete any object, the destructor is the only method to delete the object. Hence, it cannot be overloaded.
- The destructor doesn’t possess any kind of argument or any kind of return value.
- Destructor is invoked automatically when an object is going out of space.
- When a destructor is used to delete an object, the memory space of the object is also cleared which is allocated by the constructor.
- Destructors cannot be static or const.
Let’s look at a sample program implementing the destructor.
#include<iostream>
using namespace std;
class Sample
{
public:
Sample()
{
cout<<"\n Constructor of the class is executed";
}
~Sample()
{
cout<<"\n Destructor of the class is executed";
}
};
main()
{
Sample s;
return 0;
}
The output of the program is:
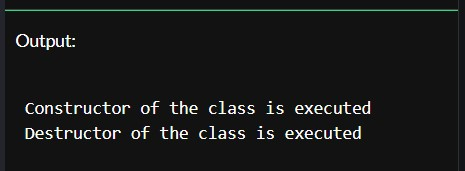
In the above program, both the constructor and the destructor of the class are defined and when an object is being defined, the constructor of the class is automatically invoked and the body of the constructor is executed. Similarly, the destructor of the class is also invoked and the body of the destructor is also executed. In this way, the constructor and the destructor of the class are implemented.
Whenever there are multiple objects are declared, the constructor is invoked for every object. The destructor is invoked in the reverse order of the constructor, which means the last object which is created is going to be deleted first, and then the order of deleting the objects followed.
Let’s understand it better by this example program.
#include<iostream>
using namespace std;
int count=0;
class Test
{
public:
Test()
{
count++;
cout<<count<<" object is created\n";
}
~Test()
{
cout<<count<<" object is destroyed\n";
--count;
}
};
main()
{
Test t,t1,t2,t3;
return 0;
}
The output of the program is.
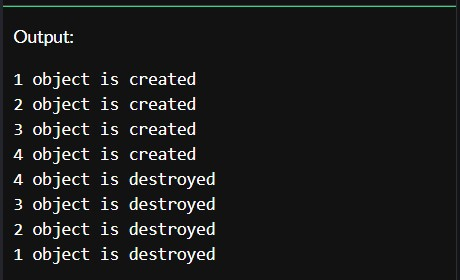
In the above program, four objects are initialized in the main method and the constructor is invoked four times for the four objects. After that, the destructor is invoked in the reverse order of the constructor, resembling the object which is created last will be deleted first, and so on.
CONCLUSION
So that’s it from this tutorial. Hope you have learned about the destructors. Happy coding!