INVALID POINTERS
A pointer is said to be an invalid pointer:
1. If it is not initialized
2. When a pointer points to a valid address but not valid elements(in arrays)
Let’s look at some examples of invalid pointers.
int *ptr1;
int arr[10];
int *ptr2 = arr+20;
In the above examples, ptr1 is declared but not initialized.
Ptr2 is assigned to arr+20, but arr has only 10 elements. Hence, ptr2 is pointing to a valid address but an invalid element.
NULL POINTERS
A pointer is said to be a NULL pointer If it is declared and initialized to 0 or NULL.
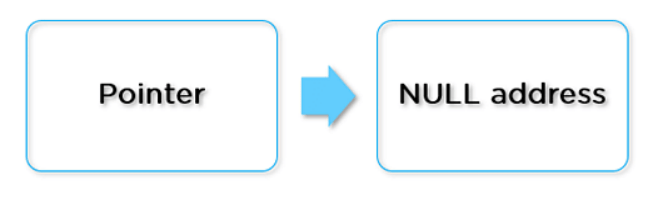
Let’s look at some examples of NULL pointers:
int *ptr1 = 0;
int *ptr2 = NULL;
WILDPOINTERS
A wild pointer is a pointer that is declared but not initialized. If we try to use the wild pointer( a pointer that is not initialized ) it may cause a segmentation fault.
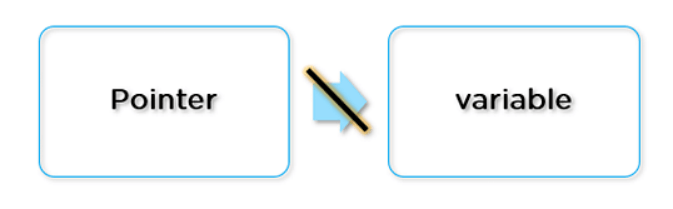
Let’s look at one example:
#include<stdio.h>
int main()
{
int *ptr;
printf(“ptr=%d”,*ptr);
return 0;
}
OUTPUT
Segmentation fault.
Dangling Pointer:
A dangling pointer is a pointer that points to a de-allocated memory location.
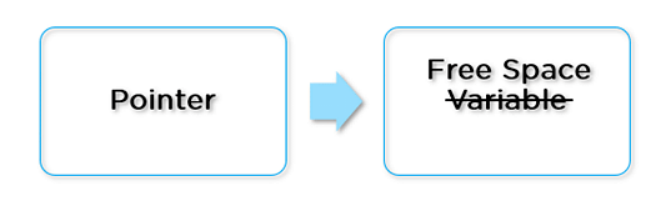
· Suppose there is a pointer p pointing at a variable at memory 1004. If you deallocate this memory, then this p is called a dangling pointer.
· You can deallocate memory using a free() function.
Miscellaneous concepts in pointers:
There are some concepts in pointers that are considered to be hard and confusing sometimes.
In this article, we are going to clarify these concepts with examples.
In this article, we will discuss the differences between constant pointers, pointers to constant & constant pointers to constants. Pointers are the variables that hold the address of some other variables, constants, or functions. There are several ways to qualify pointers using const.
· Pointers to constant.
· Constant pointers.
· Constant pointers to constant.
POINTER TO CONSTANT:
In a pointer to a constant, the data pointed by the pointer cannot be changed and is considered to be a constant.
This is the example program for the pointer to a constant:
// C++ program to illustrate concept
// of the pointers to constant
#include <iostream>
using namespace std;
// Driver Code
int main()
{
int high{ 100 };
int low{ 66 };
const int* score{ &high };
// Pointer variables are read from
// the right to left
cout << *score << "\n";
// Score is a pointer to an integer
// which is constant *score = 78
// It will give you an Error:
// assignment of read-only location
// ‘* score’ because the value stored in
// constant cannot be changed
score = &low;
// This can be done here as we are
// changing the location where the
// score points now it points to low
cout << *score << "\n";
return 0;
}
OUTPUT
100
66
Constant Pointers:
In constant pointers, the value pointed by the constant pointers can be changed. But, the pointer locates the same memory location which cannot be changed.
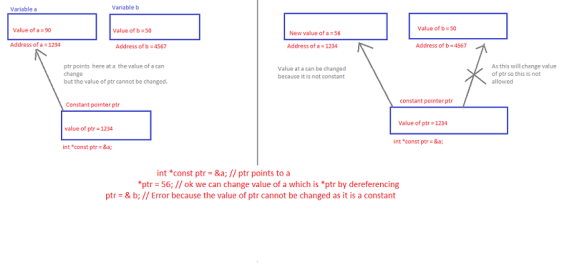
Let us look at an example:
// C++ program to illustrate concept
// of the constant pointers
#include <iostream>
using namespace std;
// Driver Code
int main()
{
int a{ 90 };
int b{ 50 };
int* const ptr{ &a };
cout << *ptr << "\n";
cout << ptr << "\n";
// Address what it points to
*ptr = 56;
// Acceptable to change the
// value of a
// Error: assignment of read-only
// variable ‘ptr’
// ptr = &b;
cout << *ptr << "\n";
cout << ptr << "\n";
return 0;
}
OUTPUT
90
0x7ffc641845a8
56
0x7ffc641845a8
CONSTANT POINTERS TO CONSTANT:
In this type of pointer, both data pointed by the pointer and the memory location cannot be changed.
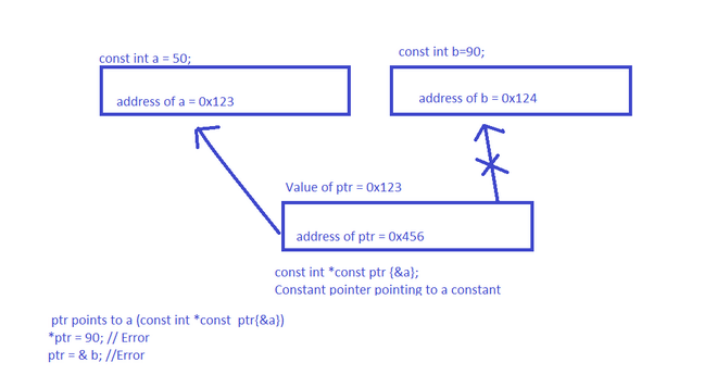
Let’s look at an example:
// C++ program to illustrate concept of
// the constant pointers to pointers
#include <iostream>
using namespace std;
// Driver Code
int main()
{
const int a{ 50 };
const int b{ 90 };
// ptr points to a
const int* const ptr{ &a };
// *ptr = 90;
// Error: assignment of read-only
// location ‘*(const int*)ptr’
// ptr = &b;
// Error: assignment of read-only
// variable ‘ptr’
// Address of a
cout << ptr << "\n";
// Value of a
cout << *ptr << "\n";
return 0;
}
OUTPUT
0x7ffea7e22d68
50
Use cases of pointers:
· Pointer arithmetic
· Pointer to pointer
· Array of pointers
· Call by value
· Call by reference
CONCLUSION:
Hurray! We have covered all the sub-topics in the pointers and to master it do some exercises on pointers. Happy Coding!