Introduction:
Text to speech is the generation of speech synthesized from the text. The technology used to communicate with users when reading on a screen is not possible or impractical. This not only opens up apps and information to use in new ways but can also make the world more accessible to people who cannot read text on a screen. In this article, I will take you through building your TTS with Python.
The technology behind the TTS has evolved over the past few decades. Using natural language processing, it is now possible to produce very natural speech that includes changes in pitch, speed, pronunciation, and inflection.
Project Prerequisites:
- We need to install several libraries for this project like – newspaper, nltk, gtts.
- We’ll also use the os module but we don’t need to install it as it is a built-in module.
- First, let’s install the newspaper library that we need. We’ll use the command – pip install newspaper3k.
- After that we’ll install the remaining modules using the command – pip install package_name.
Installing nltk
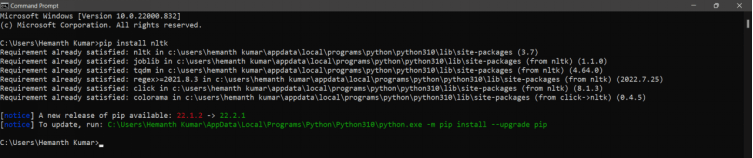
Installing gtts
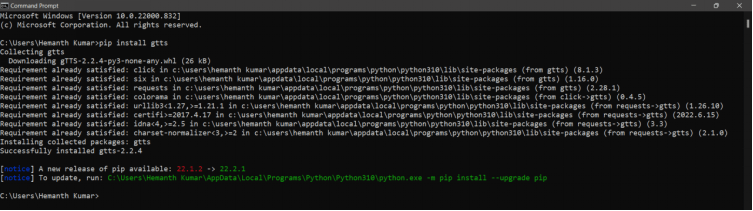
Installing the newspaper library
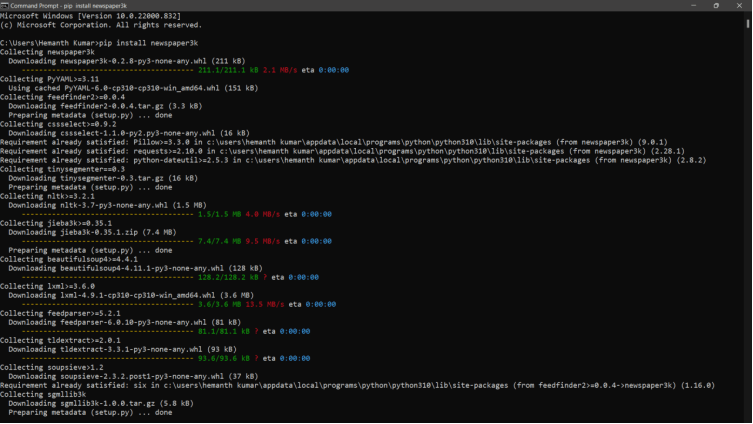
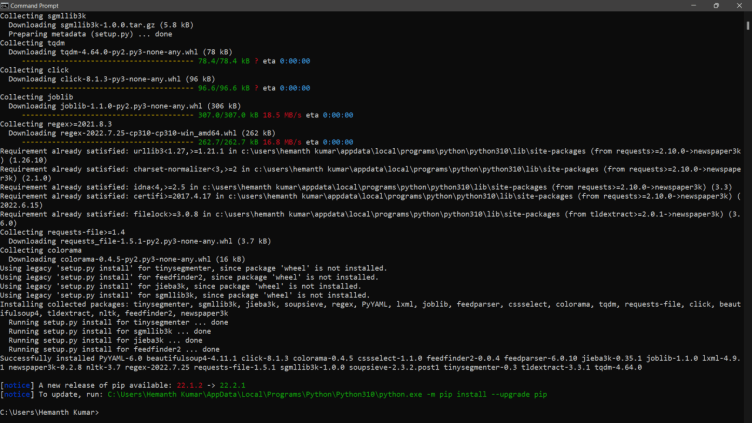
Getting Started:
First we’ll create a folder for our project named “Text_to_Speech”.
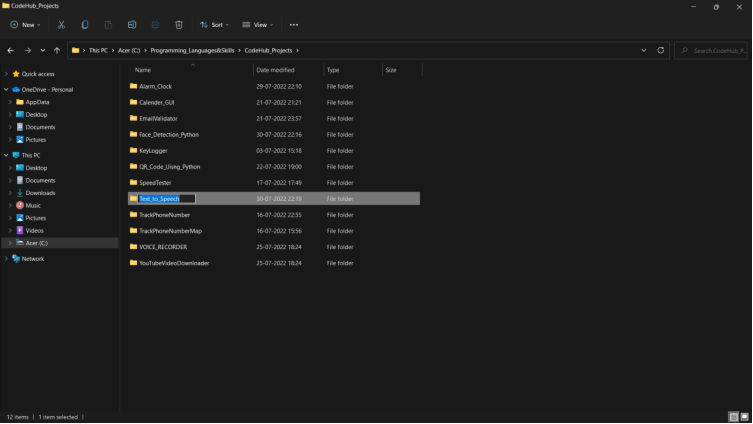
After that, let’s create a python file named “texttospeech.py”.
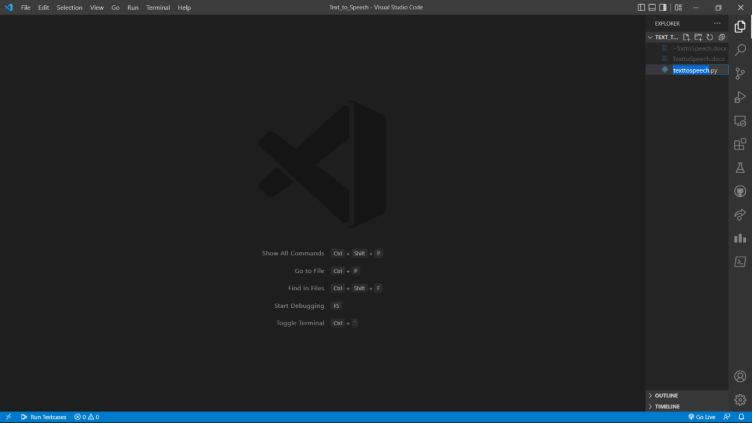
Code Implementation:
I will simply start with importing all the necessary libraries that we need for this task to create a program that takes the text of an article online and converts it into speech:
#Import the libraries
from newspaper import Article
import nltk
from gtts import gTTS
import os
#Import the libraries
from newspaper import Article
import nltk
from gtts import gTTS
import os
Now after installing and importing the libraries, we need to get an article from online sources so that we can create a program to convert text to speech from that article:
#Get the article
article = Article(‘https://hackernoon.com/future-of-python-language-bright-or-dull-uv41u3xwx’)
#Get the article
article = Article('https://hackernoon.com/future-of-python-language-bright-or-dull-uv41u3xwx')
Now, let’s download and parse the article. For this we’ll be using the .download() method and .parse() method.
article.download()
article.parse()
article.download()
article.parse()
Now you need to download the “puckt” package.
nltk.download(‘punkt’)
article.nlp()
nltk.download('punkt')
article.nlp()
Now, I will define a variable to store the article:
#Get the articles text
mytext = article.text
Now, I will define a variable to store the article:
#Get the articles text
mytext = article.text
Now we have to choose the language of speech. Note “en” means English. You can also use “pt-br” for Portuguese and there are others:
language = ‘en’ #English
language = 'en' #English
After that, we’ll need to pass the text and language to the engine to convert the text to speech and store it in a variable. Mark slow as False to tell the plug-in that the converted audio should be at high speed:
myobj = gTTS(text=mytext, lang=language, slow=False)
myobj = gTTS(text=mytext, lang=language, slow=False)
Now I’ll write the code for running text to speech.
Now, that we have converted the article for text-to-speech, so now the next step is to save this speech to mp3 file:
myobj.save(“read_article.mp3”)
myobj.save("read_article.mp3")
Now let’s play the converted audio file form text to speech in Windows, using the windows command “start” followed by the name of the mp3 file:
os.system(“start read_article.mp3”)
os.system("start read_article.mp3")
Source Code:
#Import the libraries
from newspaper import Article
import nltk
from gtts import gTTS
import os
#Get the article
article = Article(‘https://hackernoon.com/future-of-python-language-bright-or-dull-uv41u3xwx’)
article.download()
article.parse()
nltk.download(‘punkt’)
article.nlp()
#Get the articles text
mytext = article.text
language = ‘en’ #English
myobj = gTTS(text=mytext, lang=language, slow=False)
myobj.save(“read_article.mp3”)
os.system(“start read_article.mp3”)
#Import the libraries
from newspaper import Article
import nltk
from gtts import gTTS
import os
#Get the article
article = Article('https://hackernoon.com/future-of-python-language-bright-or-dull-uv41u3xwx')
article.download()
article.parse()
nltk.download('punkt')
article.nlp()
#Get the articles text
mytext = article.text
language = 'en' #English
myobj = gTTS(text=mytext, lang=language, slow=False)
myobj.save("read_article.mp3")
os.system("start read_article.mp3")
Output:
When we run the program after some time an mp3 file will be created in the same directory as the program and will be played eventually.
Note: Be patient cause this process takes time. Just sit back and wait for the audio to play.


Congratulations, now you know how to create a text-to-audio converter using python.