In this article, we will learn how to create a Ball Bouncing Game using a simple python script.
Introduction:
Let’s create a Ball Bouncing Game and make our lives a little bit easier using python.
Did anyone think about creating our program to create a Ball Bouncing Game by ourselves?
and what if I tell you!! You can do the same things with the help of a simple python script.
Outline:
In this project, we won’t take any input from the user. We just write the script and run the code. After running the script, a new window will appear showing the balls bouncing.
Project Prerequisites:
You have to install the pygame package to run this simple python script. Just we import a basic module called time and random to make use of its functions.
pygame: pygame is a Python wrapper for the SDL library, which stands for Simple DirectMedia Layer. SDL provides cross-platform access to your system’s underlying multimedia hardware components, such as sound, video, mouse, keyboard, and joystick. pygame started life as a replacement for the stalled PySDL project.
to get to know more about json, refer to: https://www.javatpoint.com/pygame
install pygame using this command:
>pip install pygame
Code Implementation:
The first thing we need to do is to import the required packages and modules. In this project, we import the pygame package, time, and random modules to make use of their functions.
import pygame, time, random
import pygame, time, random
We have to initialize the pygame by writing
pygame.init()
pygame.init()
Now, we will set the size of the window and fix the background image as backgroundimage.py attached below.
#setting screen size of pygame window to 800 by 600 pixels
screen=pygame.display.set_mode((800,600))
background=pygame.image.load(‘background-img.jpg’)


#setting screen size of pygame window to 800 by 600 pixels
screen=pygame.display.set_mode((800,600))
background=pygame.image.load('background-img.jpg')
Let’s add the title to our window.
#Adding title
pygame.display.set_caption(‘Ball Bounce Simulation’)
#Adding title
pygame.display.set_caption('Ball Bounce Simulation')
Next, we will write a class called the ball and load the ball image in it.
We will initialize all the variables using the constructor.
We will define two functions called redner_ball and move_ball
class ball:
ball_image=pygame.image.load(‘ball.png’)
g=1
def __init__(self):
self.velocityX=4
self.velocityY=4
self.X=random.randint(0,768)
self.Y=random.randint(0,350)
def render_ball(self):
screen.blit(ball.ball_image, (self.X,self.Y))
def move_ball(self):
#changing y component of velocity due to downward acceleration
self.velocityY+=ball.g
#changing position based on velocity
self.X+=self.velocityX
self.Y+=self.velocityY
#collission with the walls leads to change in velocity
if self.X<0 or self.X>768:
self.velocityX*=-1
if self.Y<0 and self.velocityY<0:
self.velocityY*=-1
self.Y=0
if self.Y>568 and self.velocityY>0:
self.velocityY*=-1
self.Y=568
class ball:
ball_image=pygame.image.load('ball.png')
g=1
def __init__(self):
self.velocityX=4
self.velocityY=4
self.X=random.randint(0,768)
self.Y=random.randint(0,350)
def render_ball(self):
screen.blit(ball.ball_image, (self.X,self.Y))
def move_ball(self):
#changing y component of velocity due to downward acceleration
self.velocityY+=ball.g
#changing position based on velocity
self.X+=self.velocityX
self.Y+=self.velocityY
#collission with the walls leads to change in velocity
if self.X<0 or self.X>768:
self.velocityX*=-1
if self.Y<0 and self.velocityY<0:
self.velocityY*=-1
self.Y=0
if self.Y>568 and self.velocityY>0:
self.velocityY*=-1
self.Y=568
In the next section, we will create 5 ball objects and store them in a list
#list of balls created as objects
Ball_List=[ball(),ball(), ball(), ball(), ball()]
#list of balls created as objects
Ball_List=[ball(),ball(), ball(), ball(), ball()]
At last, we will define a while loop to run this program for the bouncing ball to continue until we QUIT.
running=True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running=False
time.sleep(0.02)
screen.blit(background, (0,0))
for ball_item in Ball_List:
ball_item.render_ball()
ball_item.move_ball()
pygame.display.update()
running=True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running=False
time.sleep(0.02)
screen.blit(background, (0,0))
for ball_item in Ball_List:
ball_item.render_ball()
ball_item.move_ball()
pygame.display.update()
Yes. We have now completed the code implementation.
Source Code:
Here is the complete source code of the project.
#This program shows the simulation of 5 balls bouncing under gravitational acceleration.
#It is also accompanied by eleastic collission with walls of the container.
#It is fun to watch.
import pygame,time,random
pygame.init()
#setting screen size of pygame window to 800 by 600 pixels
screen=pygame.display.set_mode((800,600))
background=pygame.image.load('background-img.jpg')
#Adding title
pygame.display.set_caption('Ball Bounce Simulation')
class ball:
ball_image=pygame.image.load('ball.png')
g=1
def __init__(self):
self.velocityX=4
self.velocityY=4
self.X=random.randint(0,768)
self.Y=random.randint(0,350)
def render_ball(self):
screen.blit(ball.ball_image, (self.X,self.Y))
def move_ball(self):
#changing y component of velocity due to downward acceleration
self.velocityY+=ball.g
#changing position based on velocity
self.X+=self.velocityX
self.Y+=self.velocityY
#collission with the walls lead to change in velocity
if self.X<0 or self.X>768:
self.velocityX*=-1
if self.Y<0 and self.velocityY<0:
self.velocityY*=-1
self.Y=0
if self.Y>568 and self.velocityY>0:
self.velocityY*=-1
self.Y=568
#list of balls created as objects
Ball_List=[ball(),ball(), ball(), ball(), ball()]
#The main program loop
running=True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running=False
time.sleep(0.02)
screen.blit(background, (0,0))
for ball_item in Ball_List:
ball_item.render_ball()
ball_item.move_ball()
pygame.display.update()
As we have completed the project, let us check the output.
Output:
after running the python script like the following you will get a new window opened immediately.
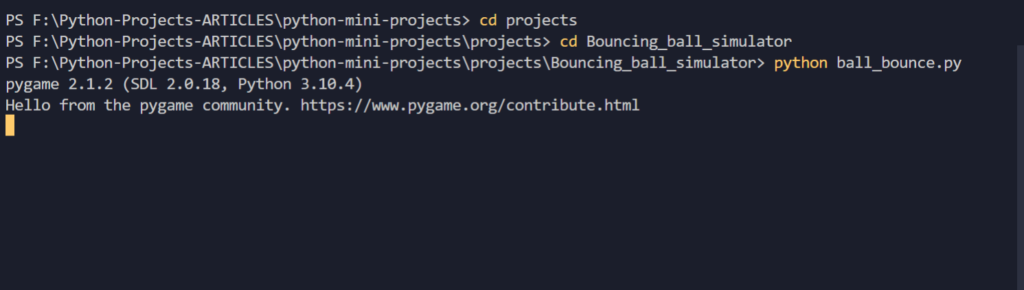
After running the source code, a new window will appear like the following:
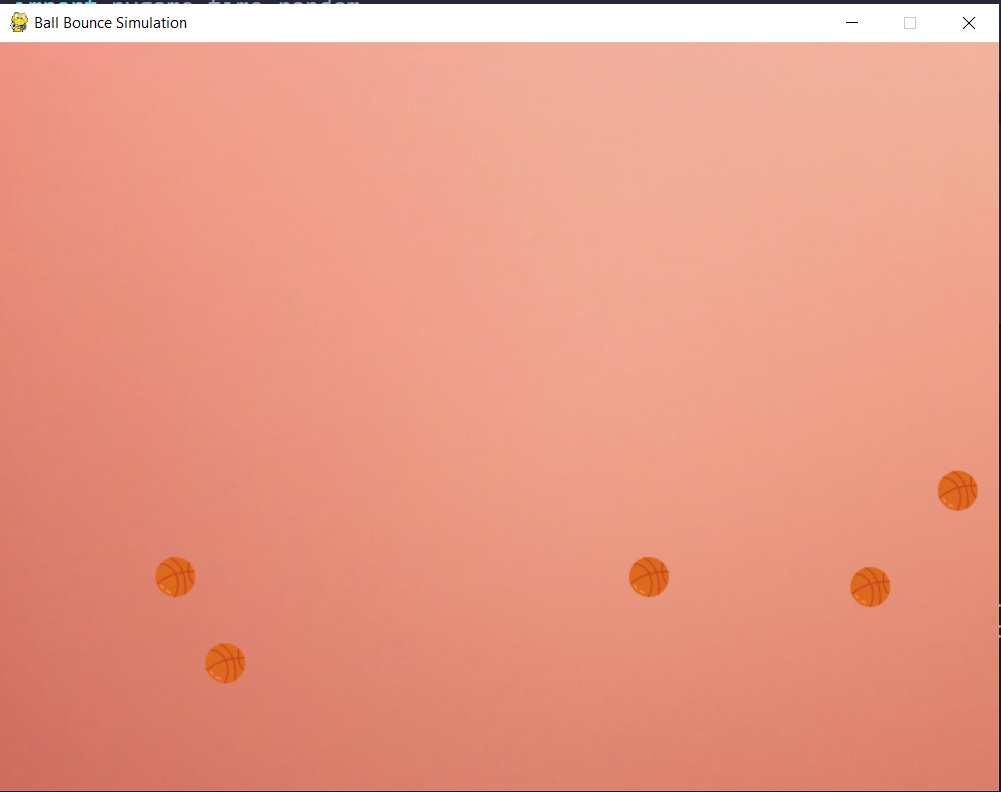
Alright, Congratulations! We have successfully learned how to create our own ball bouncing game.
Important Note:
Go to the documentation of the package: pygame package to know more about the functions used in this project.
Happy Coding.
Thank You!
By UdaySk