In this article, we will learn how to create a JSON to CSV converter using a simple python script under 15 lines of code.
Introduction:
Let’s create a JSON to CSV converter and make our lives a little bit easier using python.
Using JSON and CSV files became very important and becomes handy in our day-to-day lives. Did anyone think about creating our program to download articles as PDFs by ourselves?
and what if I tell you!! You can do the same things with the help of a simple python script.
Outline:
In this project, we will take the JSON file and give it as input for our python script and run it. After running the script we will be given/provided/created a .csv file with all the information in the JSON file.
Project Prerequisites:
You don’t have to install any package to run this simple python script. Just we import a basic package called json to make use of its functions.
json: JSON (JavaScript Object Notation), specified by RFC 7159 (which obsoletes RFC 4627) and by ECMA-404, is a lightweight data interchange format inspired by JavaScript object literal syntax (although it is not a strict subset of JavaScript 1 ).
json exposes an API familiar to users of the standard library marshal and pickle modules.
JSON JavaScript Object Notation is a format for structuring data. It is mainly used for storing and transferring data between the browser and the server. Python too supports JSON with a built-in package called json. This package provides all the necessary tools for working with JSON Objects including parsing, serializing, deserializing, and many more. Let’s see a simple example where we convert the JSON objects to Python objects and vice-versa.
to get to know more about json, refer to: https://www.geeksforgeeks.org/python-json/
Code Implementation:
The first thing we need to do is to import the required packages and modules. In this project, we import JSON package to make use of its functions.
import json
import json
Next, we will write a try block to check whether input.json file exists in the same directory.
If there is an input.json file present, We will read the data from the json file using function read() and store it in a list variable called data like the following:
try:
with open(‘input.json’, ‘r’) as f:
data = json.loads(f.read())
try:
with open('input.json', 'r') as f:
data = json.loads(f.read())
In the next section, we define another variable called output and store all the information that we fetched from the input.json file.
output = ‘,’.join([*data[0]])
for obj in data:
output += f’\n{obj[“Name”]},{obj[“age”]},{obj[“birthyear”]}’
output = ','.join([*data[0]])
for obj in data:
output += f'\n{obj["Name"]},{obj["age"]},{obj["birthyear"]}'
Now, we will open/create an output.csv file and write all the contents/data present in our output variable.
with open(‘output.csv’, ‘w’) as f:
f.write(output)
with open('output.csv', 'w') as f:
f.write(output)
At last, we will define an exception block and print the error.
except Exception as ex:
print(f’Error: {str(ex)}’)
except Exception as ex:
print(f'Error: {str(ex)}')
Yes. We have now completed the code implementation.
Source Code:
Here is the complete source code of the project.
import json
if __name__ == ‘__main__’:
try:
with open(‘input.json’, ‘r’) as f:
data = json.loads(f.read())
output = ‘,’.join([*data[0]])
for obj in data:
output += f’\n{obj[“Name”]},{obj[“age”]},{obj[“birthyear”]}’
with open(‘output.csv’, ‘w’) as f:
f.write(output)
except Exception as ex:
print(f’Error: {str(ex)}’)
import json
if __name__ == '__main__':
try:
with open('input.json', 'r') as f:
data = json.loads(f.read())
output = ','.join([*data[0]])
for obj in data:
output += f'\n{obj["Name"]},{obj["age"]},{obj["birthyear"]}'
with open('output.csv', 'w') as f:
f.write(output)
except Exception as ex:
print(f'Error: {str(ex)}')
As we have completed the project, let us check the output.
Output:
After running the below-given command, make sure you are running the file from a correct directory.
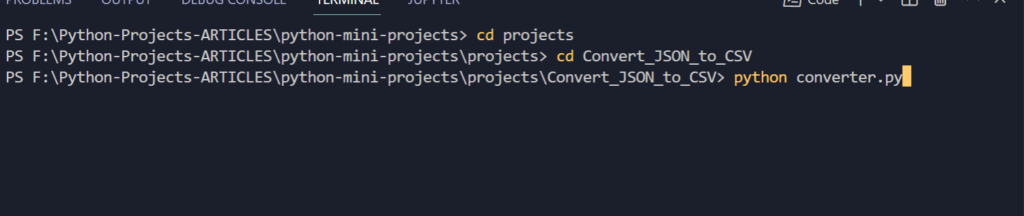
Run the python script like the above-given command.
After running the source code, the output.csv file will be created and the information will be there like the following example:

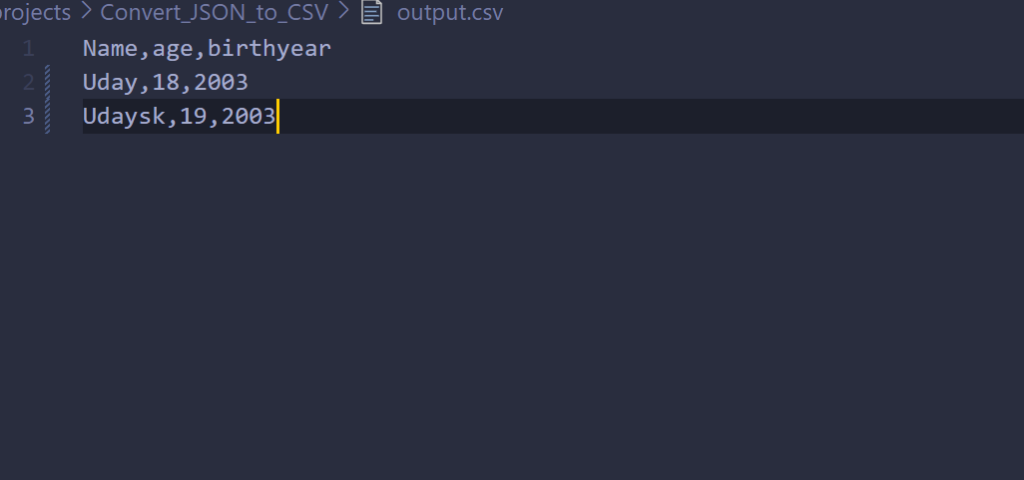
Congratulations! You have created a JSON to CSV converter using a simple python script.
Important Note:
To get to know more about CSV and JSON, just search about it or drop a comment below.
Happy Coding.
Thank You!
By UdaySk