If you are procrastinating reading your favorite book because you are a little lazy. Then no worries being a programmer, you can make Python read a book for you as well. Not just an ebook but any pdf or text can be converted into audio within just 12 lines of Python Coding. So now you can even lay down on your bed and listen to your favorite book. Follow these steps properly to convert your eBook into an Audiobook.
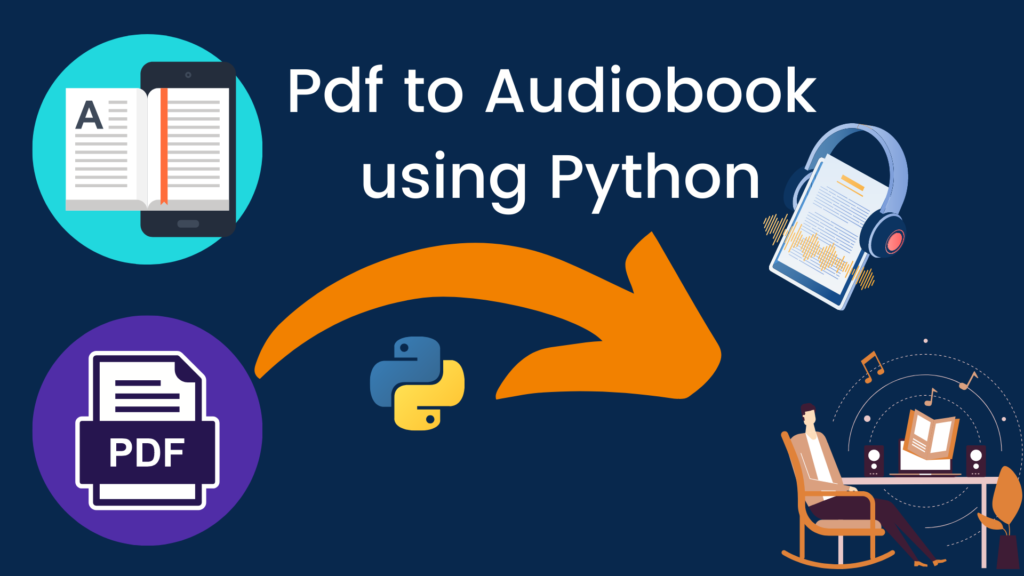
Step 1 Installing Requirements
So to get started you need the following things:
Python IDLE: If you don’t have Python installed in your system, you can go to the download section in python.org and get it from there.
Pycharm: The second thing you need is your code editor. Where you can write and execute your code. For this, I’ll use Pycharm. This you can get on the official website of Pycharm. It has two versions Professional and Community. The community version is free whereas the Professional version is paid one. Here I’m using the community version from their website.
So once I install pycharm I’ll create a new project and will save it into my PycharmProjects folder. Inside that location, I’ll give it a name as Pdf to Audiobook. After setting its location and giving it a name just click on Create at the bottom right corner.

This may take from a few seconds up to a minute for getting ready all the environment and packages. Once the project is ready I need to create a new file from the file menu. I’ll give it a name main and press enter.

To check whether the file is ready or not you can type print(‘Anything’) and try to run it. So for that right-click on it and then click on the option Run file in the Python Console. If that’s successfully executed then you will see ‘Anything’ here:


So that means our First Step is now complete. We have Python installed, Pycharm Installed and we can successfully run our code.
Step 2 Importing Libraries
Comming to step number two our primary job is to make our code speak. So for that, we need to install a python package. Just go to the terminal option at the bottom of your pycharm window and type there: pip install pyttsx3. That means I’m telling pip to install a package called as pyttsx3 (Python text to speech) which is a text to speech converter library in Python.

Based on your system speed this might take around a minute to install this python text to speech package. Let it be done.
After that you just need four lines of coding to make your system speak:
import pyttsx3
speaker = pyttsx3.init()
speaker.say('Hey look I can speak')
speaker.runAndWait()
Basically, the text quoted as ‘Hey look I can speak’ is the actual text that it will read. This is just simple as that. To execute or run this code you can tap on the play button at the top right corner. Then you can your system speaking. So you are done with step number 2.
Step 3 Extracting Pdf
In step number 3 our task is to get a pdf file and extract the number of pages into it. After that, we’ll look into how to make Python read the text or content from that Pdf. So in this step, you need a pdf that you want python to read for you. If it is an ebook then convert it into pdf format. I’m using XII BST Case Studies-ch-2.pdf.
Now if you are downloading the pdf or getting it from any other source you have to save it into the file “Pdf to Audiobook” created by us in the first step. If this has been saved successfully there, then I’ll see it next to my main.py file in Pycharm.

Now as I have a pdf file, I need another python package to read the pdf file. So again to install this we will go to the terminal and type there: pip install PyPDF2 and press enter. Once you have it you need to do a few more work:
import PyPDF2
book = open('XII BST Case Studies-ch-2.pdf', 'rb')
pdfReader = PyPDF2.PdfFileReader(book)
pages = pdfReader.numPages
print(pages)
The above part of the code will come here:
import pyttsx3
import PyPDF2
book = open('XII BST Case Studies-ch-2.pdf', 'rb')
pdfReader = PyPDF2.PdfFileReader(book)
pages = pdfReader.numPages
print(pages)
speaker = pyttsx3.init()
speaker.say('Hey listen I can speak')
speaker.runAndWait()
If you will run the above code you can see the number of pages in the console. Which will be exactly the same as that was in your pdf.
Step 4 Making Python Read
Moving ahead to our next step we will now look into how to make it read the desired page from our pdf. Till now we have got a pdf reader and a speaker that can read anything given to it. Now to initialize this speaker and read a single page from our pdf we will take a variable ‘page‘:
speaker = pyttsx3.init()
page = pdfReader.getPage()
In the empty () you can put the page number you want it to read. Remember if we want to read page 10 we need to put 9 because in the programming index the number starts from 0.
And to extract text from the variable we need another variable:
speaker = pyttsx3.init()
page = pdfReader.getPage(9)
text = page.extractText()
After getting the text extracted you have to add this ‘text‘ replacing the previous ‘Hey listen I can speak‘ into the speaker:
speaker = pyttsx3.init()
page = pdfReader.getPage(9)
text = page.extractText()
speaker.say(text)
That’s it!
By this now you can convert any pdf or ebook into Audiobook. After this, your python will read the book for you.
Complete Code
Here’s the complete code that we had learned in the above steps. In the code below you just have to change the name of pdf (abcd) and the page number (6) and the Python will covert your eBook or pdf into Audiobook. So enjoy listening.
import pyttsx3
import PyPDF2
book = open('abcd', 'rb')
pdfReader = PyPDF2.PdfFileReader(book)
pages = pdfReader.numPages
print(pages)
speaker = pyttsx3.init()
page = pdfReader.getPage(6)
text = page.extractText()
speaker.say(text)
speaker.runAndWait()
Check more such amazing Python Projects by us.