Making projects is always the best way of excelling in a programming language. In this article, I’m going to share with you a Fidget Spinner game project using Python, with complete source code and resources. From this project, you will get some ideas to level up your python learning skills. Moreover, you can add this python project to your resume or portfolio. So, let’s start looking into the steps of making our Fidget Spinner.
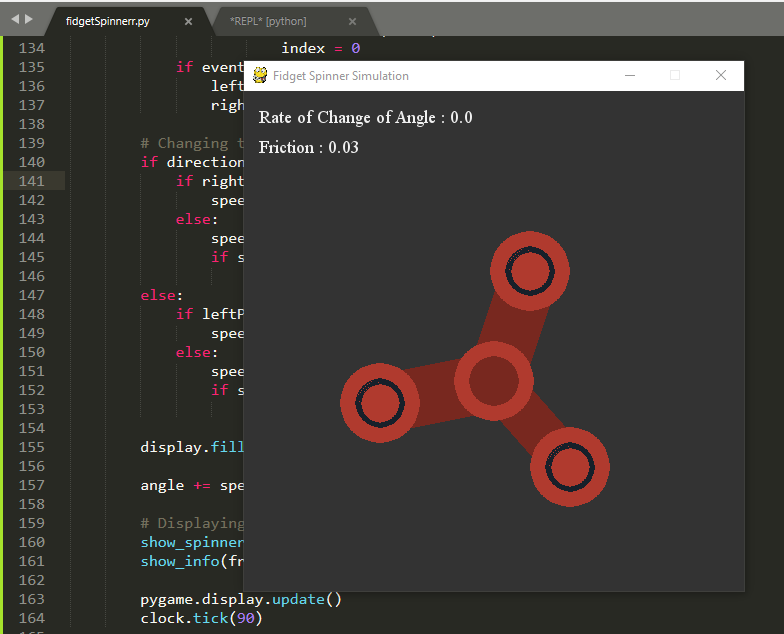
Required Modules
Before looking at the code make sure that you have installed the required modules.
math – For doing maths for rotation
sys – We will use sys to exit the program
pygame – To build 2D games in Python
You can tap on the above links, attached with their respective names to know furthermore about them.
Library Installation
So before getting started with the code, we need to install the necessary required module or library. As by default random and sys comes preinstalled in python, we only need to install pygame. And for doing that, you need to write the following command in your terminal or command prompt.
pip install pygame
Code
Now comes the code.
import pygame
import sys
from math import *
importing the required modules. which is necessary otherwise, you will encounter errors.
# Initialization of Pygame Window
pygame.init()
width = 500
height = 500
display = pygame.display.set_mode((width, height))
clock = pygame.time.Clock()
pygame.display.set_caption("Fidget Spinner Simulation")
# Colors
background = (51, 51, 51)
white = (240, 240, 240)
red = (176, 58, 46)
dark_red = (120, 40, 31)
dark_gray = (23, 32, 42)
blue = (40, 116, 166)
dark_blue = (26, 82, 118)
yellow = (183, 149, 11)
dark_yellow = (125, 102, 8)
green = (29, 131, 72)
dark_green = (20, 90, 50)
orange = (230, 126, 34)
dark_orange = (126, 81, 9)
# Close the Pygame Window
def close():
pygame.quit()
sys.exit()
In this part of the code, I have just initialized the pygame and set the value like width and height of the pygame window. besides setting the value of the pygame window I have defined the value of RGB color in tuples. and lastly, I have also written the program to close the window.
# Drawing of Fidget Spinner on Pygame Window
def show_spinner(angle, color, dark_color):
d = 80
innerd = 50
x = width/2 - d/2
y = height/2
l = 200
r = l/(3**0.5)
w = 10
lw = 60
# A little math for calculation the coordinates after rotation by some 'angle'
# x = originx + r*cos(angle)
# y = originy + r*sin(angle)
centre = [x, y, d, d]
centre_inner = [x + d/2 - innerd/2, y + d/2 - innerd/2, innerd, innerd]
top = [x, y - l/(3)**0.5, d, d]
top_inner = [x, y - l/(3)**0.5, innerd, innerd]
top[0] = x + r*cos(radians(angle))
top[1] = y + r*sin(radians(angle))
top_inner[0] = x + d/2 - innerd/2 + r*cos(radians(angle))
top_inner[1] = y + d/2 - innerd/2 + r*sin(radians(angle))
left = [x - l/2, y + l/(2*(3)**0.5), d, d]
left_inner = [x, y - l/(3)**0.5, innerd, innerd]
left[0] = x + r*cos(radians(angle - 120))
left[1] = y + r*sin(radians(angle - 120))
left_inner[0] = x + d/2 - innerd/2 + r*cos(radians(angle - 120))
left_inner[1] = y + d/2 - innerd/2 + r*sin(radians(angle - 120))
right = [x + l/2, y + l/(2*(3)**0.5), d, d]
right_inner = [x, y - l/(3)**0.5, innerd, innerd]
right[0] = x + r*cos(radians(angle + 120))
right[1] = y + r*sin(radians(angle + 120))
right_inner[0] = x + d/2 - innerd/2 + r*cos(radians(angle + 120))
right_inner[1] = y + d/2 - innerd/2 + r*sin(radians(angle + 120))
# Drawing shapes on Pygame Window
pygame.draw.line(display, dark_color, (top[0] + d/2, top[1] + d/2), (centre[0] + d/2, centre[1] + d/2), lw)
pygame.draw.line(display, dark_color, (left[0] + d/2, left[1] + d/2), (centre[0] + d/2, centre[1] + d/2), lw)
pygame.draw.line(display, dark_color, (right[0] + d/2, right[1] + d/2), (centre[0] + d/2, centre[1] + d/2), lw)
pygame.draw.ellipse(display, color, tuple(centre))
pygame.draw.ellipse(display, dark_color, tuple(centre_inner))
pygame.draw.ellipse(display, color, tuple(top))
pygame.draw.ellipse(display, dark_gray, tuple(top_inner), 10)
pygame.draw.ellipse(display, color, tuple(left))
pygame.draw.ellipse(display, dark_gray, tuple(left_inner), 10)
pygame.draw.ellipse(display, color, tuple(right))
pygame.draw.ellipse(display, dark_gray, tuple(right_inner), 10)
Now I have drawn the fidget spinner on the pygame window with the help of the pygame draw function, as a name said draw() used to draw shapes and lines on the pygame window. With all A little math for calculation the coordinates after rotation by some ‘angle’.
# Displaying Information on Pygame Window
def show_info(friction, speed):
font = pygame.font.SysFont("Times New Roman", 18)
frictionText = font.render("Friction : " + str(friction), True, white)
speedText = font.render("Rate of Change of Angle : " + str(speed), True, white)
display.blit(speedText, (15, 15))
display.blit(frictionText, (15, 45))
This block is code used to show information like speeds etc on the pygame window with setting up the properties of the custom font.
# The Main Function
def spinner():
spin = True
angle = 0
speed = 0.0
friction = 0.03
rightPressed = False
leftPressed = False
direction = 1
color = [[red, dark_red], [blue, dark_blue], [yellow, dark_yellow], [green, dark_green], [orange, dark_orange]]
index = 0
while spin:
for event in pygame.event.get():
if event.type == pygame.QUIT:
close()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
close()
if event.key == pygame.K_RIGHT:
rightPressed = True
direction = 1
if event.key == pygame.K_LEFT:
leftPressed = True
direction = -1
if event.key == pygame.K_SPACE:
index += 1
if index >= len(color):
index = 0
if event.type == pygame.KEYUP:
leftPressed = False
rightPressed = False
# Changing the Angle of rotation
if direction == 1:
if rightPressed:
speed += 0.3
else:
speed -= friction
if speed < 0:
speed = 0.0
else:
if leftPressed:
speed -= 0.3
else:
speed += friction
if speed > 0:
speed = 0.0
display.fill(background)
angle += speed
# Displaying Information and the Fidget Spinner
show_spinner(angle, color[index][0], color[index][1])
show_info(friction, speed)
pygame.display.update()
clock.tick(90)
spinner()
Here in this block of code main loop of the game is written. and also set the value of angle, speed, and rotation of the fidget spinner. after setting these values a while loop is used for continuous rotation of the fidget spinner and also certain keys are defined for rotation for a fidget spinner. each time the button has clicked the value of rotation and speeds also increased.
Here is complete Source Code
import pygame
import sys
from math import *
pygame.init()
width = 500
height = 500
display = pygame.display.set_mode((width, height))
clock = pygame.time.Clock()
pygame.display.set_caption("Fidget Spinner Simulation")
# Colors
background = (51, 51, 51)
white = (240, 240, 240)
red = (176, 58, 46)
dark_red = (120, 40, 31)
dark_gray = (23, 32, 42)
blue = (40, 116, 166)
dark_blue = (26, 82, 118)
yellow = (183, 149, 11)
dark_yellow = (125, 102, 8)
green = (29, 131, 72)
dark_green = (20, 90, 50)
orange = (230, 126, 34)
dark_orange = (126, 81, 9)
# Close the Pygame Window
def close():
pygame.quit()
sys.exit()
# Drawing of Fidget Spinner on Pygame Window
def show_spinner(angle, color, dark_color):
d = 80
innerd = 50
x = width/2 - d/2
y = height/2
l = 200
r = l/(3**0.5)
w = 10
lw = 60
# A little math for calculation the coordinates after rotation by some 'angle'
# x = originx + r*cos(angle)
# y = originy + r*sin(angle)
centre = [x, y, d, d]
centre_inner = [x + d/2 - innerd/2, y + d/2 - innerd/2, innerd, innerd]
top = [x, y - l/(3)**0.5, d, d]
top_inner = [x, y - l/(3)**0.5, innerd, innerd]
top[0] = x + r*cos(radians(angle))
top[1] = y + r*sin(radians(angle))
top_inner[0] = x + d/2 - innerd/2 + r*cos(radians(angle))
top_inner[1] = y + d/2 - innerd/2 + r*sin(radians(angle))
left = [x - l/2, y + l/(2*(3)**0.5), d, d]
left_inner = [x, y - l/(3)**0.5, innerd, innerd]
left[0] = x + r*cos(radians(angle - 120))
left[1] = y + r*sin(radians(angle - 120))
left_inner[0] = x + d/2 - innerd/2 + r*cos(radians(angle - 120))
left_inner[1] = y + d/2 - innerd/2 + r*sin(radians(angle - 120))
right = [x + l/2, y + l/(2*(3)**0.5), d, d]
right_inner = [x, y - l/(3)**0.5, innerd, innerd]
right[0] = x + r*cos(radians(angle + 120))
right[1] = y + r*sin(radians(angle + 120))
right_inner[0] = x + d/2 - innerd/2 + r*cos(radians(angle + 120))
right_inner[1] = y + d/2 - innerd/2 + r*sin(radians(angle + 120))
# Drawing shapes on Pygame Window
pygame.draw.line(display, dark_color, (top[0] + d/2, top[1] + d/2), (centre[0] + d/2, centre[1] + d/2), lw)
pygame.draw.line(display, dark_color, (left[0] + d/2, left[1] + d/2), (centre[0] + d/2, centre[1] + d/2), lw)
pygame.draw.line(display, dark_color, (right[0] + d/2, right[1] + d/2), (centre[0] + d/2, centre[1] + d/2), lw)
pygame.draw.ellipse(display, color, tuple(centre))
pygame.draw.ellipse(display, dark_color, tuple(centre_inner))
pygame.draw.ellipse(display, color, tuple(top))
pygame.draw.ellipse(display, dark_gray, tuple(top_inner), 10)
pygame.draw.ellipse(display, color, tuple(left))
pygame.draw.ellipse(display, dark_gray, tuple(left_inner), 10)
pygame.draw.ellipse(display, color, tuple(right))
pygame.draw.ellipse(display, dark_gray, tuple(right_inner), 10)
# Displaying Information on Pygame Window
def show_info(friction, speed):
font = pygame.font.SysFont("Times New Roman", 18)
frictionText = font.render("Friction : " + str(friction), True, white)
speedText = font.render("Rate of Change of Angle : " + str(speed), True, white)
display.blit(speedText, (15, 15))
display.blit(frictionText, (15, 45))
# The Main Function
def spinner():
spin = True
angle = 0
speed = 0.0
friction = 0.03
rightPressed = False
leftPressed = False
direction = 1
color = [[red, dark_red], [blue, dark_blue], [yellow, dark_yellow], [green, dark_green], [orange, dark_orange]]
index = 0
while spin:
for event in pygame.event.get():
if event.type == pygame.QUIT:
close()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
close()
if event.key == pygame.K_RIGHT:
rightPressed = True
direction = 1
if event.key == pygame.K_LEFT:
leftPressed = True
direction = -1
if event.key == pygame.K_SPACE:
index += 1
if index >= len(color):
index = 0
if event.type == pygame.KEYUP:
leftPressed = False
rightPressed = False
# Changing the Angle of rotation
if direction == 1:
if rightPressed:
speed += 0.3
else:
speed -= friction
if speed < 0:
speed = 0.0
else:
if leftPressed:
speed -= 0.3
else:
speed += friction
if speed > 0:
speed = 0.0
display.fill(background)
Credits and notes
Fidget Spinner Simulation
- Language: Python
- Modules: Pygame, sys, math
- Controls : It has the following controls:
- Firstly, Left and Right arrows to rotate the Spinner in direction,
- Secondly, Spacebar to change color of Spinner,
- And finally, the longer you hold down the arrow key, the higher its speed.
So, the above project was inspired by Jatin Kumar Yadav. Hope so that the above project Fidget Spinner using Python could have been helpful to you. If so then you can also check Space Shooter Game, Flappy Bird Game, 2D Snake Game, Notepad, etc. using Python on this page.
If you want to explore more contents and projects by Jatin Kumar Yadav the following links can help you:
Website: https://jatinmandav.wordpress.com
YouTube Channel: https://www.youtube.com/channel/UCdpf6Lz3V357cIZomPwjuFQ
Facebook: https://www.facebook.com/jatinmandav
Twitter: @jatinmandav
Email: jatinmandav3@gmail.com