In this article, we are going to learn about Inheritance in detail.
Introduction:
Inheritance is a mechanism that allows us to inherit all the properties from another class. Acquiring base class properties and methods into a child class is known as inheritance. The main advantage of this is re-usability and increases readability. Python supports single-level, multi-level and multiple inheritances. In python, the object is the parent class of all classes.
It generally means “inheriting or transfer of characteristics from parent to child class without any modification”. The new class is called the derived /child class and the one from which it is derived is called a parent/base class.
Python inheritance super function – super().
With inheritance, the super() function in python actually comes in quite handy. It allows us to call a method from the parent class.
Python super() function provides us the facility to refer to the Parent class explicitly. It is basically useful where we have to call super class functions. It returns the proxy object that allows us to refer to the parent class by “super”.
Different forms of inheritances:
- Single level inheritance
- Multi-level inheritance
- Multiple inheritance
- Hierarchical inheritance
Single inheritance:
Single-level inheritance enables a derived class to inherit characteristics from a single parent class. When a child class inherits from only one parent, it is called single inheritance
Single-level inheritance enables code reusability of a parent class, and adding new features to a class makes code more readable, elegant, and less redundant. And thus, single-level inheritance is much safer than multiple inheritances if it’s done in the right way and enables the derived class to call the parent class method and also override the parent class’s existing methods.
Sample Diagram for Single Inheritance:
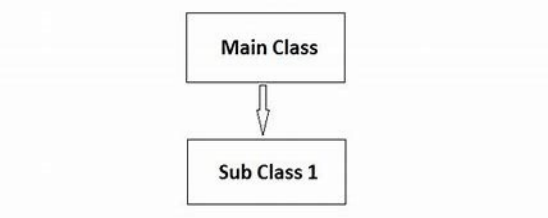
Syntax for single inheritance:
class super_class:
#block of code of super class....
class child_class(parent_class):
#block of code of child class......
Example:
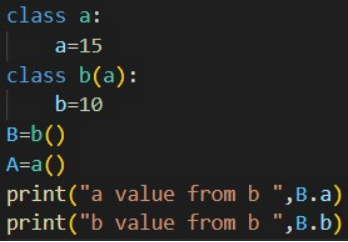
Output:

Multi-level inheritance:
When one class inherits another, which in turn inherits from another, it is called as multi-level inheritance. Multi-level inheritance enables a derived class to inherit properties from an immediate parent class which in turn inherits properties from his parent class.
Sample Diagram for multi-level Inheritance:
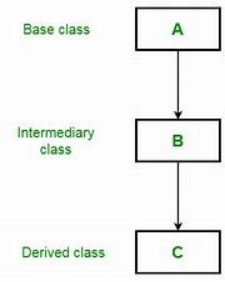
Syntax for multi-level inheritance:
class super_class1:
#block of code of super class 1....
class derived_class(super_class1):
#block of code of derived class......
class derived_class1(derived_class):
#block of code of derived class1
Example:
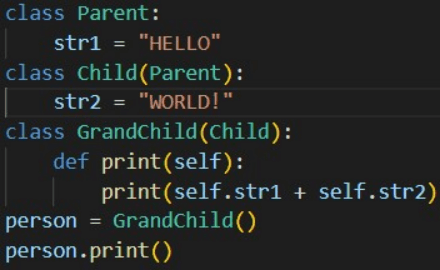
Output:

Multiple inheritance:
Multiple python inheritance is when a class inherits from multiple base classes. when a child class inherits from multiple parent classes, it is called multiple inheritance. Multiple level inheritance enables one derived class to inherit properties from more than one base class.
Sample diagram for multiple inheritance:
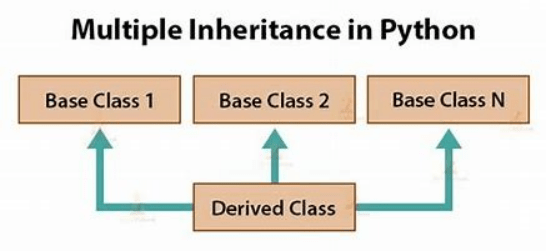
Syntax for multiple inheritance:
class super_class1:
#block of code of super class1.....
class super_class2:
#block of code of super class2....
class derived_class(super_class1 , super_class2):
#block of code of derived class......
Example:
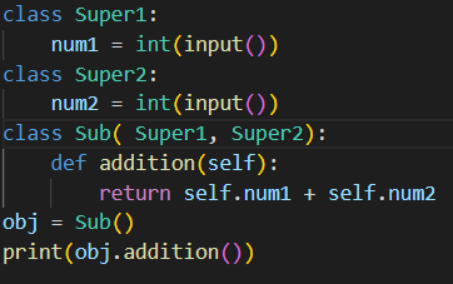
Output:
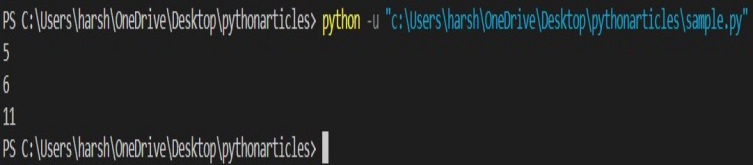
Hierarchical inheritance:
Hierarchical level inheritance enables more than one derived class to inherit properties from a parent class. When more than one class inherits from a class, it is known as hierarchical inheritance.
Sample diagram for hierarchical inheritance:
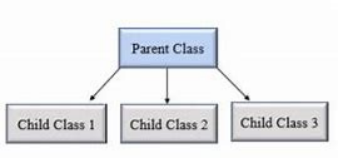
Syntax for hierarchical inheritance:
class derived_class2(super_class):
#block of code of derived_class2…
Example:
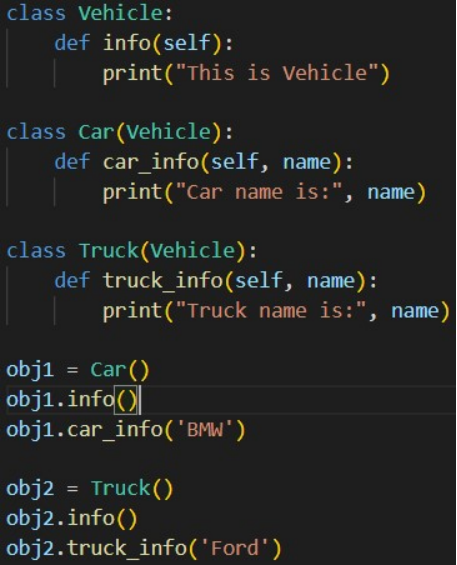
Output:
